In this C programming class, we’ll cover the C decision-making constructs such as C if, if-else, and the switch-case statement. It is also known as conditional programming in C.
In your life, you encounter situations where you have to make a decision be it your favorite food dish or the color of your new car. In C programming also, you may encounter such situations where you need to make a decision.
The conditions in C language will help you. C primarily provides the following three types of conditional or decision-making construct.
Don’t miss out on how to write your first C program in just 2 minutes.
If Statement in C
The if statement helps you check a particular condition. If that condition is true, then a specific block (enclosed under the if) of code gets executed.
This flowchart will help you.
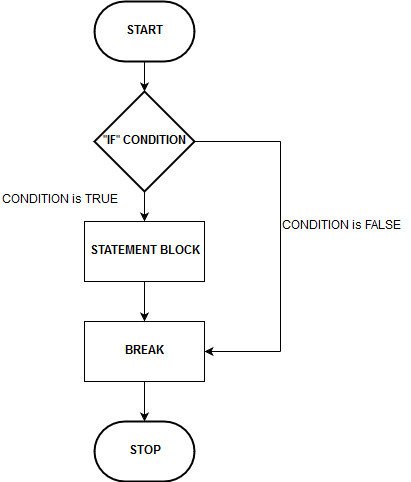
C If Syntax:
if(condition either true or false) { //Statement block }
Now we will see a simple program using an if statement.
Program using C If Statement to Find the Greatest of Two Numbers.
Flowchart:
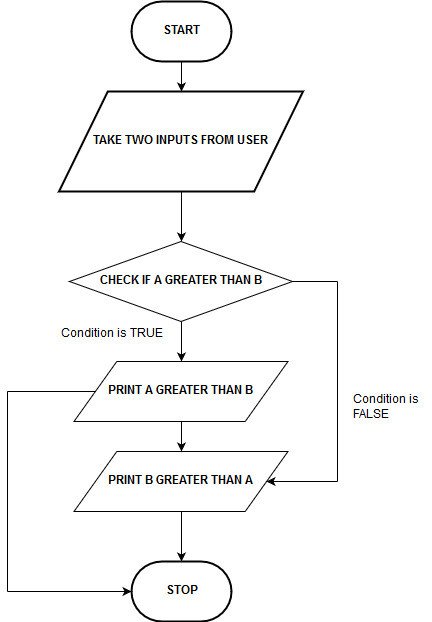
Algorithm:
Step 1: Start. Step 2: Take two inputs (a and b) from the user. Step 3: If a is greater than b then go to step 4 otherwise go to step 5 Step 4: Print a greater than b Step 5: Print b greater than a Step 6: Stop.
Code:
#include<stdio.h> #include<conio.h> void main() { int a,b; printf("Enter two numbers :"); scanf("%d %d",&a,&b); if (a>b) printf("%d is greater",a); printf("%d is greater",b); getch(); }
The output is as follows:
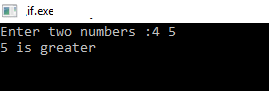
If…Else Statement in C
The if statement works pretty well, but if you want to work with more variables and more data, the if-else statement comes into play.
In the if statement, only one block of code executes after the condition is true.
But in the if-else statement, there are two blocks of code – one for handling the success and the other for the failure condition.
This flowchart will help you get it.
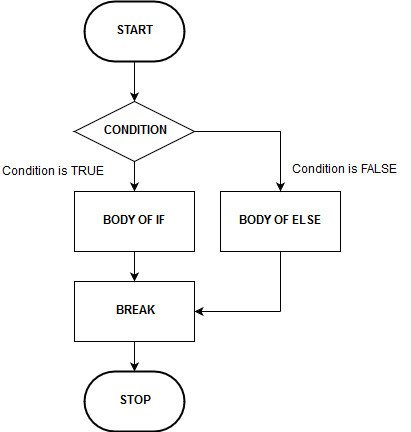
C If…Else Syntax:
if(condition) { //Statement block } else { //Statement block }
Program using C If…Else to Find Whether a Number is Odd or Even.
Flowchart:
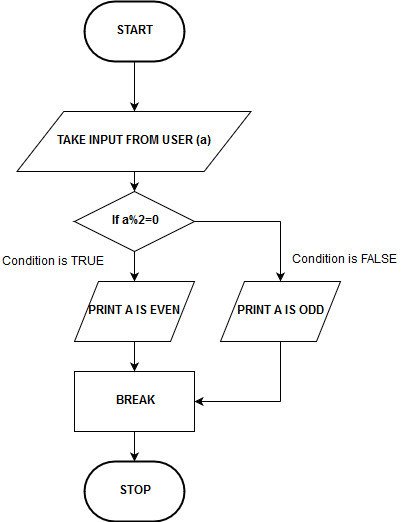
Algorithm:
Step 1: Start. Step 2: Take input from the user. Step 3: Check condition. If remainder is zero go to step 4 else go to step 5 Step 4: Print a is even and go to step 6 Step 5: Print a is odd Step 6: Stop
Code:
#include<stdio.h> #include<conio.h> void main() { int a; printf("Enter a number :"); scanf("%d",&a); if (a%2==0) { printf("%d is even",a); } else printf("%d is odd",a); getch(); }
The output should look something like this-
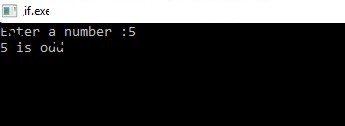
You can use multiple if-else statements which get called “nested if-else” statements. It is no different than the one above you can use various if-else statements in this order. Just keep in mind that the sequence should end with a final else statement, not an if statement.
Switch-Case Statement in C
When you have to execute multiple statements under one operation, Switch-case comes into play.
There are several cases under one switch statement.
C Switch…Case Syntax:
switch(variable) { case n1: //Statement block; break; case n2: //Statement block; break; . . . case n: //Statement block; break; }
Here the variable is taken from the user as input.
Program using C Switch…Case to Calculate the Area of a Rectangle/Circle/Triangle.
Flowchart:
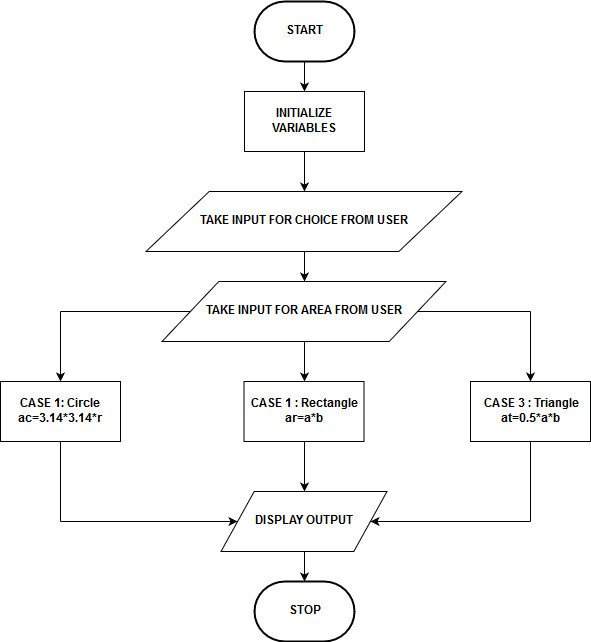
Algorithm:
Step 1: Start Step 2: Initialize variables Step 3: Take input for choice and then for area variables from the user Step 4: Case 1: Circle: 3.14*3.14*r Case 2: Rectangle: ar=a*b Case 3: Triangle: at=0.5*a*b Step 5: Display output according to case Step 6: Stop
Code:
#include<stdio.h> #include<conio.h> void main() { int ac,ar,at,r,a,b,choice; printf("Enter your choice\n”); prinft(“A for area of circle\n”); printf(“B for area of rectangle\n”); printf(“C for area of triangle\n"); scanf("%c",&choice); switch(choice) { case A: printf("Enter radius: "); scanf("%d",&r); ac=3.14*3.14*r; printf("Area of circle is: %d",ac); break; case B: printf("Enter length and breadth:"); scanf("%d %d",&a,&b); ar=a*b; printf("Area of rectangle is: %d",ar); break; case C: printf("Enter base and height: "); scanf("%d %d",&a,&b); at=0.5*a*b; printf("Area of triangle is: %d",at); break; } getch(); }
Output:
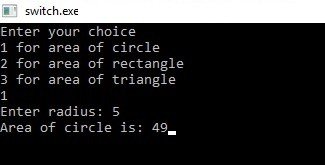