📝 Check out a comprehensive set of Matplotlib exercises and practice with our Online Matplotlib Compiler. This library is mainly used for data visualization in Python. From this tutorial, you will get some idea about – how to analyze trends, build machine learning models, and explore datasets.
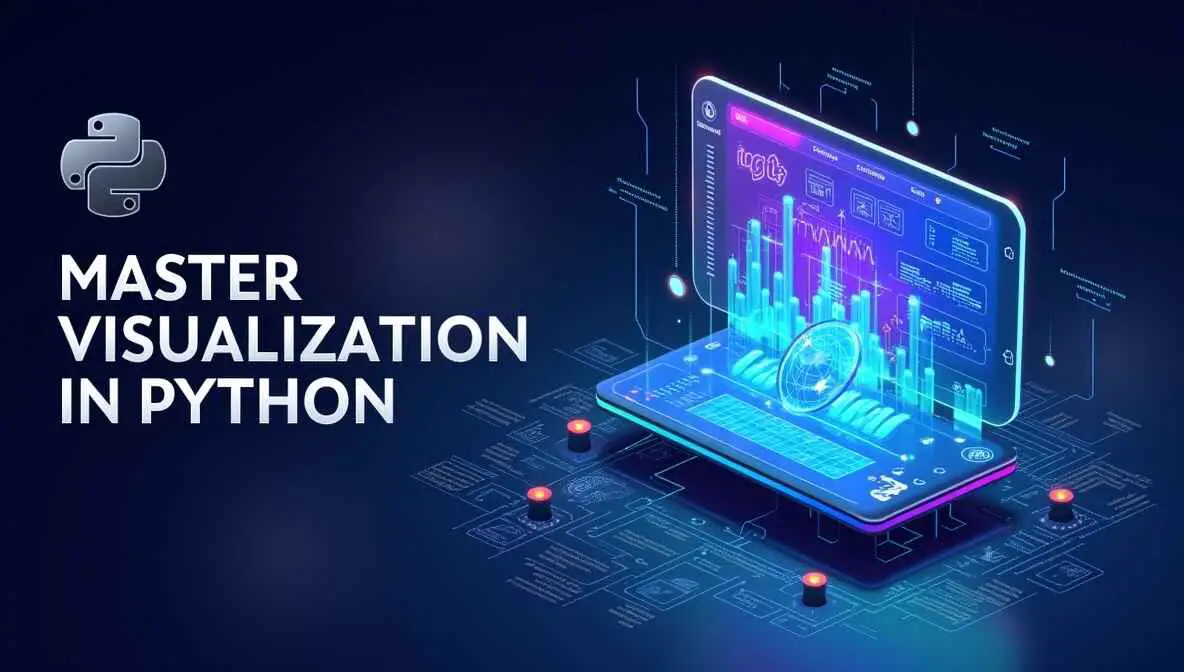
What is Matplotlib?
Matplotlib is famous for its data visualizing features. It comes as a package for Python. It includes several plot types such as bar charts, scatter plots, histograms, pie, and various other. This is how, it helps us in analyzing data, in machine learning, and helping us understand trends and patterns easily.
We have included many practical exercises in this tutorial to demonstrate the usage of Matplotlib. To compile and run them, you will need to install Python 3 and Matplotlib package.
However, with an online Matplotlib compiler, you can write, test, and visualize data in seconds. No setup. No delays. Just pure coding. Let’s dive in!
Understanding How Matplotlib Plots Work
Every Matplotlib visualization consists of these core components. It is important to know these before start to dive into the exercises.
Matplotlib Core Components | Description |
---|---|
Figure Canvas | The “blank page” where your plot lives Created automatically when you import matplotlib.pyplot |
Axes | The actual plotting area (where lines/bars appear) Contains the x-axis and y-axis |
Data Layer | Your actual plot (lines, bars, dots) Added via commands like plot() , bar() , scatter() |
Annotations | Text elements: titles, axis labels, legends Added with title() , xlabel() , ylabel() |
Types of Plots in Matplotlib
The following few are a basic and commonly used plot type:
- Bar Charts (Great for comparisons)
- Scatter Plots (For correlations)
- Histograms (Data distribution)
- Pie Charts (Proportions & percentages)
Why Use an Online Matplotlib Compiler?
Matplotlib is the #1 Python library for data visualization, used in analytics, machine learning, and research. But setting it up locally can be tedious.
With our free online Matplotlib compiler, you can:
✔ Plot instantly – No installations or setup.
✔ Practice anywhere – Works on all devices.
✔ Access key libraries – Matplotlib, pandas, and NumPy pre-installed.
✔ Learn faster – Experiment without breaking your local environment.
Ideal for:Â Data scientists, students, and developers who need quick, hassle-free plotting.
Practical Matplotlib Exercises (Try Them Live!)
Let’s now learn how to use Matplotlib in Python. Please ensure either you have opened our online matplotlib compiler or press the “run code” button in the top left of the coding snippets.
Exercise 1: Your First Plot – Website Traffic Trend
The purpose of this example is to make you aware of the Matplotlib core components. We’ll create a simple line plot showing monthly website visitors. Here’s what each element does:
import matplotlib.pyplot as plt # The visualization engine
# Sample data
months = ["Jan", "Feb", "Mar", "Apr"] # X-axis values
visitors = [1200, 1800, 2100, 1600] # Y-axis values
# Creating the plot
plt.plot(months, visitors,
color="blue", # Line color
marker="o", # Data point markers
linestyle="--") # Dashed line
# Adding labels and title
plt.title("Monthly Website Visitors") # Chart title
plt.xlabel("Month") # X-axis label
plt.ylabel("Visitors") # Y-axis label
# Enhancing readability
plt.grid(True) # Show grid lines
plt.show() # Display the plot
👉 Key Learning Points
The code created the following line plot.
plt.plot()
 – Creates the basic line chart- Customization options (color, marker, linestyle)
- Essential labels for clarity
- Grid lines for better data interpretation
Try modifying:
- RemoveÂ
linestyle
 to get a solid line - ChangeÂ
color="green"
 to see immediate effect - Try different markers:Â
"s"
 (square),Â"^"
 (triangle)
Exercise 2: Product Comparison – Bar Chart
With this exercise, you’ll learn when to use the bar chart. It is perfect for comparing discrete categories. We’ll visualize quarterly product sales:
Example: Bar Chart
import matplotlib.pyplot as plt
products = ["Laptops", "Phones", "Tablets"] # Categories
sales = [200, 350, 150] # Values
plt.bar(products, sales,
color=["#4CAF50", "#2196F3", "#FF5722"], # Custom colors
width=0.6) # Bar width
plt.title("Q1 Product Sales")
plt.ylabel("Units Sold (Thousands)")
plt.ylim(0, 400) # Setting Y-axis range
plt.show()
👉 What’s different here?
You can see that three bars are formed in our bar chart, each reflecting a product.
plt.bar()
 instead ofÂplot()
 for categorical data- Custom color palette using hex codes
ylim()
 to control axis rangewidth
 parameter adjusting bar thickness
Pro Tip: Add this line before show()
 to display exact values on bars:
for i, v in enumerate(sales):
plt.text(i, v+10, str(v), ha='center')
Exercise 3: Data Relationships – Scatter Plot
This exercise show cases a basic correlation between two variables. Let’s examine ad spend vs. revenue:
import matplotlib.pyplot as plt
ad_spend = [100, 200, 300, 400] # X-axis
revenue = [150, 350, 420, 500] # Y-axis
plt.scatter(ad_spend, revenue,
color="red",
s=100) # Marker size
plt.title("Ad Spend vs. Revenue")
plt.xlabel("Advertising Budget ($)")
plt.ylabel("Revenue Generated ($)")
plt.show()
👉 Analysis Techniques
- Positive correlation? Points moving upwards right
- Outliers? Points far from the general cluster
- No correlation? Randomly scattered points
Enhancement:Â Add a trendline with:
import numpy as np
z = np.polyfit(ad_spend, revenue, 1)
p = np.poly1d(z)
plt.plot(ad_spend, p(ad_spend), "b--")
Exercise 4: Data Distribution – Histogram
They show how numerical data is distributed – crucial for statistics and machine learning pre-processing.
import matplotlib.pyplot as plt
ages = [22, 45, 30, 34, 28, 40, 35, 29, 33, 27, 31, 38]
plt.hist(ages,
bins=5, # Number of bars
color="purple",
edgecolor="black", # Bar borders
alpha=0.7) # Transparency
plt.title("Customer Age Distribution")
plt.xlabel("Age Groups")
plt.ylabel("Number of Customers")
plt.show()
👉 Interpreting OUR Histogram Results
i. Skewed Left/Right? Our data shows:
- A short “tail” on the right (ages 44.4-50 has just 1 customer)
- Most data clusters on the left side (ages 22-38.8)
This means:
- Our customer base is younger-skewed
- The 45-year-old is an outlier compared to others
ii. Normal Distribution? Our plot is NOT perfectly normal because:
- No clear bell curve shape
- Peaks at 27.6-33.2 range (5 customers)
- Missing middle-aged customers (38.8-44.4 group is empty)
iii. Bins Matter!
With bins=5
:
âś…Â Good:Â Clearly shows:
- The 27.6-33.2 age group dominates
- The 44.4-50 group is a clear outlier
❌ If you changed bins:
bins=2
: Would hide the empty 38.8-44.4 groupbins=10
: Might show empty bins between ages
🎓 Take It Further: Advanced Practice Ideas
✔ Add annotations (plt.annotate()
) to highlight key data points.
✔ Use subplots (plt.subplots()
) for side-by-side comparisons.
✔ Try themes (plt.style.use('ggplot')
) for professional styling.
🎯 Ready to Try It Yourself?
Click here to open our Online Matplotlib Compiler and run these examples live!
💬 Which Matplotlib plot will you try first? Drop a comment below! 👇
Final Thoughts
An online Matplotlib compiler removes barriers, letting you focus on what matters—mastering data visualization. Whether you’re a student, data analyst, or Python enthusiast, practicing online accelerates learning.
🚀 Happy Plotting!
🔗 Share this guide with fellow coders who need a quick way to practice Matplotlib!