A for loop is a basic tool for performing iterative tasks. This tutorial covers the Python for loop syntax, flowchart, and multiple variations with examples. This makes it easy for you to learn loops and use them in your Python programs.
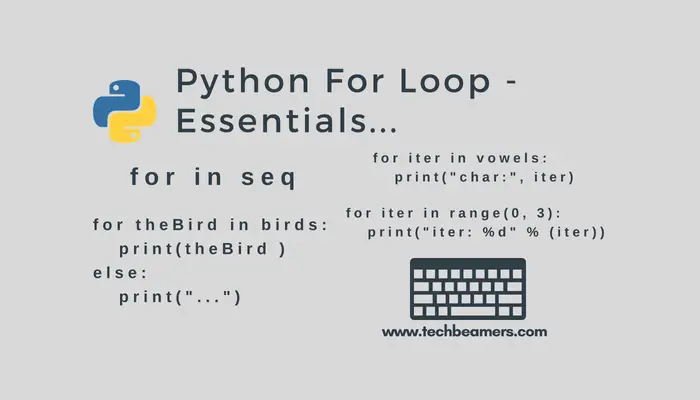
How to Use For Loops in Python
Python provides a simple syntax for using for loops in programs. They help iterate through different types of objects. Python supports seven sequence data types: standard/Unicode strings, lists, tuples, byte arrays, and range objects. Sets and dictionaries also exist, but they are unordered collections rather than sequence types.
Python For Loop Syntax
A for loop in Python requires at least two variables to work. The first is an iterable object, such as a list, tuple, or string. The second is a variable that stores successive values from the sequence. Check its syntax below:
# Syntax: Python for loop
for iter in sequence:
statements(iter)
- The “iter” represents the iterating variable. It gets assigned successive values from the input sequence.
- The “sequence” may refer to any of the following Python objects such as a list, a tuple, or a string.
Python For Loop Flowchart
The for loop can include a single line or a block of code with multiple statements. Before executing the code inside the loop, the value from the sequence gets assigned to the iterating variable (“iter”).
Below is the For loop flowchart representation in Python:
Python For Loop: Multiple Variations with Examples
You can use a for loop to iterate through various data types in Python. Let’s go through them one by one
Python For Loop with Strings, Lists, and Dictionaries
Let’s start with a simple example: printing all characters in a Python string using a for loop.
# Program to print the letters of a string
vowels = "AEIOU"
for iter in vowels:
print("char:", iter)
""" Output
char: A
char: E
char: I
char: O
char: U
"""
Now, we’ll see how to use the for loop with a Python list. To demonstrate, we’ll use a list of N numbers and compute their average.
# Program to calculate the average of N integers int_list = [1, 2, 3, 4, 5, 6] sum = 0 for iter in int_list: sum += iter print("Sum =", sum) print("Avg =", sum/len(int_list))
Here is the output after executing the above code.
""" Output Sum = 21 Avg = 3.5 """
Moving on, let’s see how a for loop works with nested lists. The code below demonstrates how to print each element of a nested list in Python.
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
for row in matrix:
for element in row:
print(element, end=" ")
print()
Next, let’s see how to iterate over a dictionary in Python.
# A dictionary with mixed data types as keys
mix = {
"string": 123,
100: "value",
(1, 2): [True, False],
None: "null"
}
# Iterate over the dictionary using enumerate()
for index, (key, value) in enumerate(mix.items()):
print(f"Item {index + 1}: Key = {key}, Value = {value}")
Python For Loop with range(), zip(), and enumerate()
The Python range() function generates a sequence of numbers at runtime.
For example, a statement like range(0, 10) will generate a series of ten integers starting from 0 to 9. The snippet below shows how range() works and how you can access its elements by index.
Note: In Python 3, range() returns a range object, not a list. You can convert it to a list if needed.
# Check the type of list returned by range() function
print( type(range(0, 10)) )
# <class 'range'>
# Access the first element in the range sequence
print( range(0, 10)[0] ) # 0
# Access the second element
print( range(0, 10)[1] ) # 1
# Access the last element
print( range(0, 10)[9] ) # 9
# Calculate the size of range list
print( len(range(0, 10)) ) # 10
Now, let’s see how range() works inside a for loop.
for iter in range(0, 3): print("iter: %d" % (iter))
It will yield the following result.
""" Output iter: 0 iter: 1 iter: 2 """
By default, the for loop fetches elements from the sequence and assigns them to the iterating variable. But you can also make it return the index by replacing the sequence with a range(len(seq)) expression.
books = ['C', 'C++', 'Java', 'Python'] for index in range(len(books)): print('Book (%d):' % index, books[index])
The following lines will get printed.
""" Output Book (0): C Book (1): C++ Book (2): Java Book (3): Python """
The Python zip() function lets you iterate over multiple lists simultaneously, pairing items based on their position.
list1 = [1, 2, 3]
list2 = ["a", "b"]
for x, y in zip(list1, list2):
print(x, y) # Output: 1 a, 2 b
Next, let’s see how the Python enumerate() helps when iterating with a for loop.
mix = ["hello", 42, True, (1, 2), None]
for index, element in enumerate(mix):
print(f"Item {index + 1}: {element}")
More Python For Loop Examples
For loop with else clause
Interestingly, Python allows an optional else statement with the for loop. The code inside the else clause runs only if the loop completes without encountering a break statement.
Check the below syntax to use the else clause with the loop.
for item in seq:
statement 1
statement 2
if <condition>: # If this condition is met, loop breaks
break
else:
statements # Runs only if loop completes normally
Check out the flowchart below to understand how the else clause works with a for loop.
Below is the sample code that uses a for loop and an else statement.
# Demonstrating else with for loop
birds = ['Belle', 'Coco', 'Juniper', 'Lilly', 'Snow']
ignore_else = False # Improved variable name for clarity
for bird in birds:
print(bird)
if ignore_else and bird == 'Snow':
break
else:
print("No birds left.")
The code prints all bird names and, if the loop runs fully, it executes the else block.
Nested for loops
A nested for loop is a loop inside another loop. It’s useful for iterating over multi-dimensional data, like lists of lists.
for i in range(5):
for j in range(3):
if i == 2 and j == 1:
break
print(i, j)
For loop with pass
A pass statement inside a for loop does nothing – it acts as a placeholder when you need a loop structure but don’t want to execute any code.
for num in range(10):
if num % 2 == 0:
continue # Skip even numbers
pass # Placeholder statement
print(num)
For loop with break
A break statement stops a for loop before it completes all iterations. It is useful when you want to exit the loop based on a condition.
numbers = [1, 2, 3, 4, 5]
for num in numbers:
if num > 3:
break # Exit loop when num > 3
print(num)
For loop in one line
A Python for loop can be written in a single line for conciseness. This is useful for simple operations on each element in a sequence.
for num in range(10): print(f"Number {num}: {num ** 2}")
Summary: Python For Loop with Examples
In this tutorial, we explained the concept of Python for Loop with several examples so that you can easily use it in real-time Python programs. However, if you have any questions about this topic, please do write to us.
Enjoyed this tutorial? Support us by subscribing to our YouTube channel and sharing this post on LinkedIn or Twitter! Your support helps us keep creating free, high-quality content.
Happy Coding,
TechBeamers.