This guide teaches you how to write a unique and engaging Python game code, we call it Neon Racer. The focus is on smooth gameplay, clear instructions, and a modular approach. Follow along to understand how each piece (such as setting up the window, player movement, obstacles, pickups, scoring, and game over conditions) fits into the complete project.

Get Started with Neon Race Python Game Code
Neon Racer is a fast-paced arcade game built with Python and Pygame. In this game, you control a neon vehicle racing on a scrolling road. Your main objective is to avoid falling obstacles while collecting pickups that boost your score. The game demonstrates key aspects of python game code, including:
• Setting up a game window with a steady frame rate
• Implementing smooth player movement
• Randomly generating obstacles
• Detecting collisions to end the game
• Tracking the player’s score
Now, it’s time to follow this step-by-step Python guide to create the Neon Racer game.
Install and Set Up Pygame
First, ensure you have Python installed. Then install Pygame via the terminal:
pip install pygame
Test your installation with:
import pygame
print(pygame.__version__)
This confirms your system is ready to run python codes for games.
Step-1: Creating the Game Window
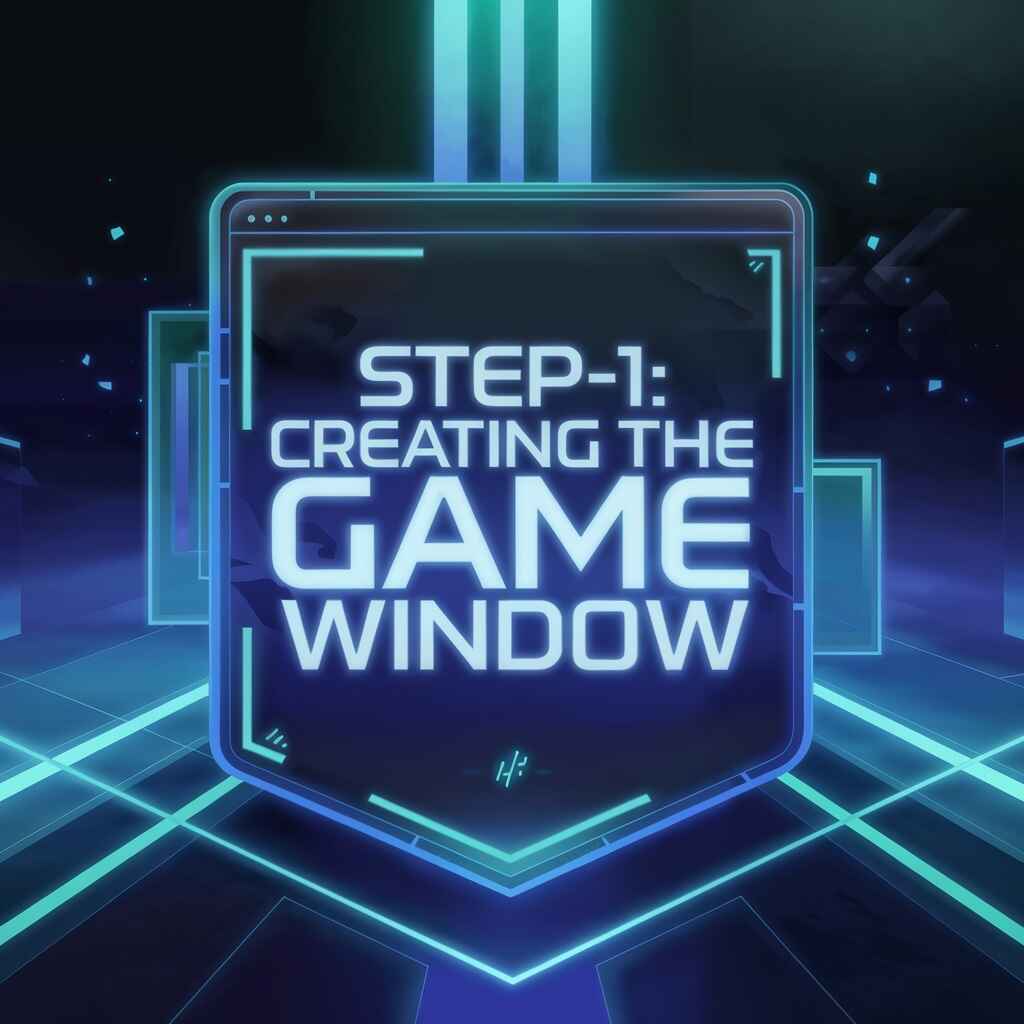
Your game window is the canvas for Neon Racer. Create a window with a consistent frame rate using this module:
import pygame
pygame.init()
WIDTH, HEIGHT = 800, 600
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Neon Racer")
clock = pygame.time.Clock()
# This code sets up a smooth 60 FPS window, serving as the base for your game code in python.
Test this part separately—when you run it, a window should open.
Step-2: Implement Player Movement
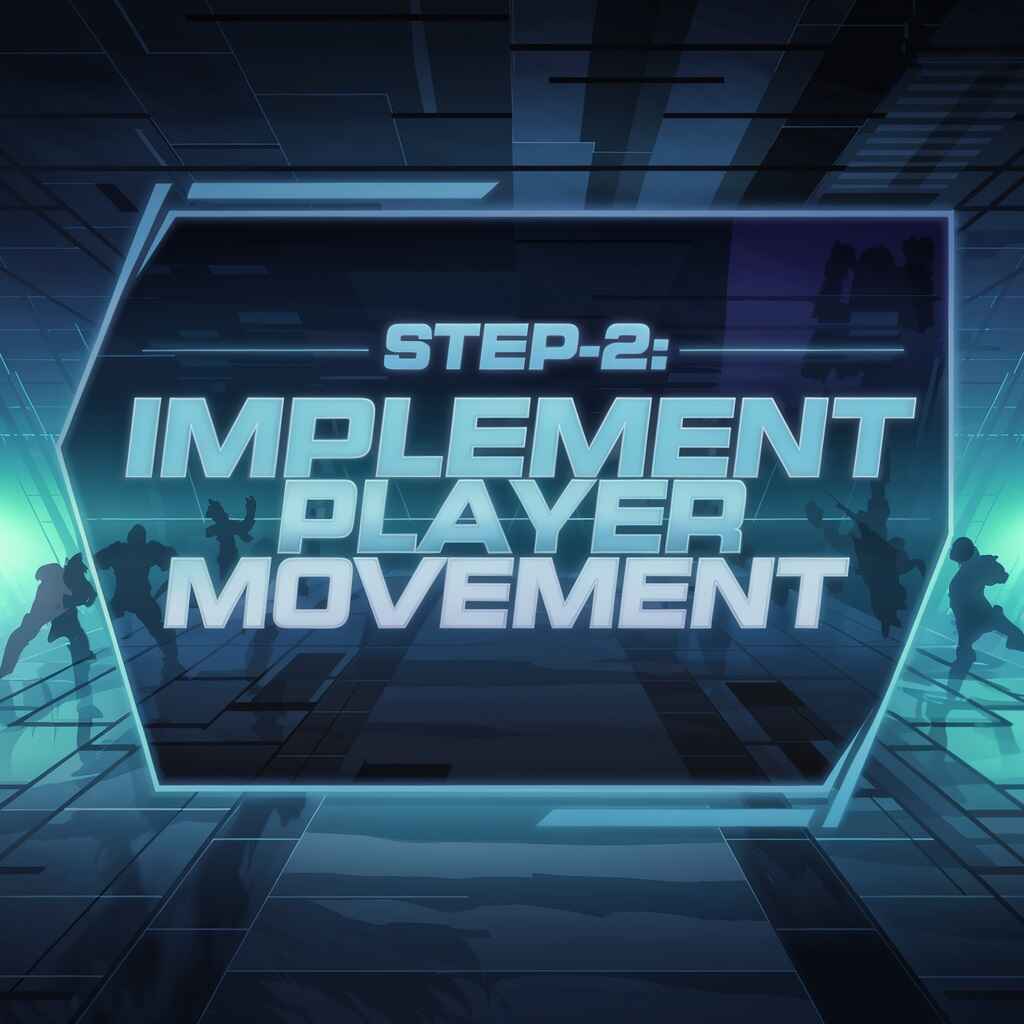
In Neon Racer, the player controls a neon vehicle. This section teaches you how to set up smooth left/right movement.
# Initialize player properties
player_x = WIDTH // 2
player_y = HEIGHT - 120
player_speed = 7
def move_player(keys, x):
if keys[pygame.K_LEFT] and x > 0:
x -= player_speed
if keys[pygame.K_RIGHT] and x < WIDTH - 60:
x += player_speed
return x
# To test, call move_player inside your game loop and draw a rectangle representing the vehicle:
# pygame.draw.rect(screen, (0, 255, 255), (player_x, player_y, 60, 30))
After integrating, test the movement to ensure your neon vehicle responds to arrow keys.
Step-3: Add a Scrolling Road Background
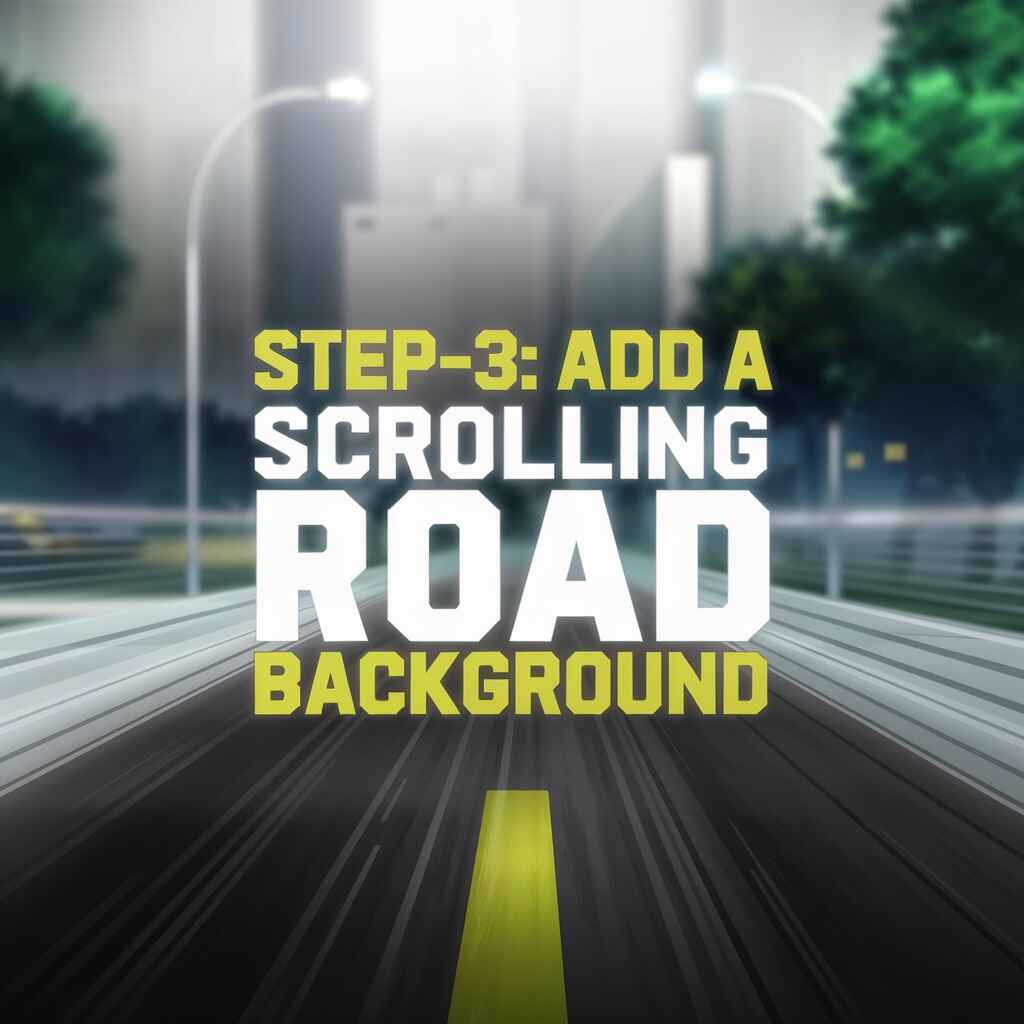
To give the game a dynamic feel, add a scrolling background that simulates a moving road.
# Create two background rectangles that scroll down continuously.
road_y = 0
road_speed = 5
def update_road(y, speed):
y += speed
if y >= HEIGHT:
y = 0
return y
# In your game loop, update road_y and draw two rectangles:
# pygame.draw.rect(screen, (50, 50, 50), (200, y, 400, HEIGHT))
# pygame.draw.rect(screen, (50, 50, 50), (200, y - HEIGHT, 400, HEIGHT))
This module is a “puzzle piece” that, when added, provides a sense of movement and depth.
Step-4: Generating Obstacles
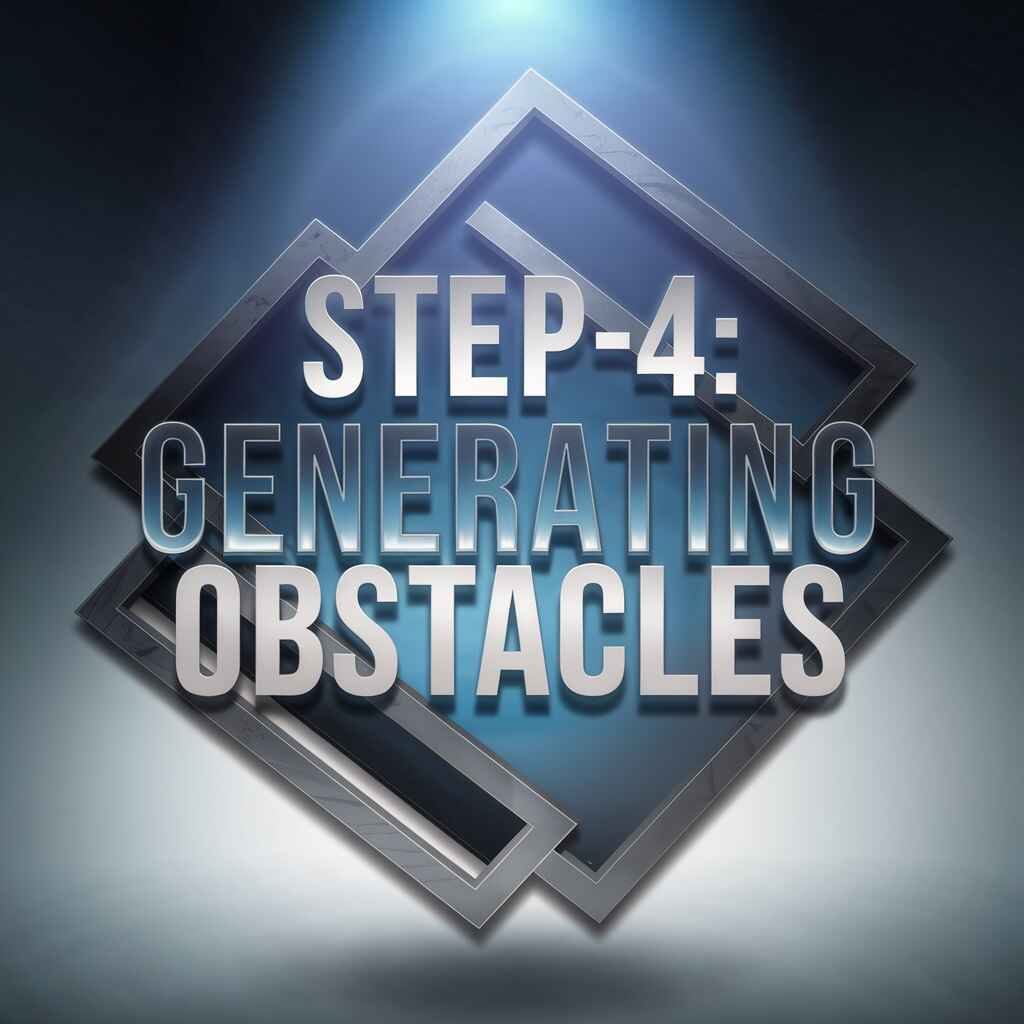
Neon Racer challenges you to dodge obstacles. These obstacles are generated at random positions at the top and fall toward the player.
import random
obstacles = []
obstacle_spawn_rate = 30 # frames
obstacle_speed = 4
def spawn_obstacle():
x = random.randint(220, 800 - 220) # keeping obstacles within the road boundaries
y = -50
speed = random.randint(4, 7)
obstacles.append([x, y, speed])
def update_obstacles(obstacles, current_speed=1.0):
for obstacle in obstacles[:]:
obstacle[1] += obstacle[2] * current_speed
if obstacle[1] > HEIGHT:
obstacles.remove(obstacle)
return obstacles
Test this module separately to ensure obstacles appear and move down.
Step-5: Add Pickups for Extra Points
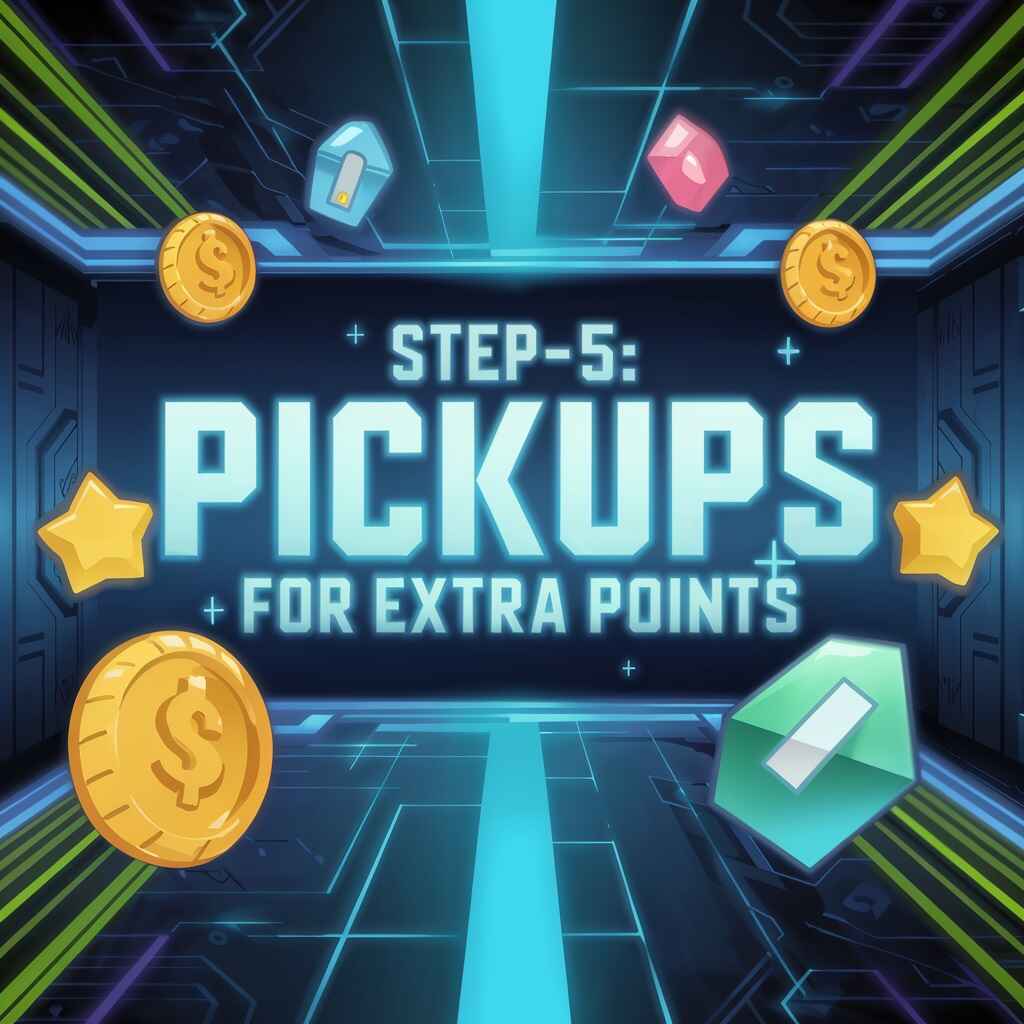
To make the game more engaging, add pickups that the player can collect. These boost the score or give temporary advantages.
pickups = []
pickup_spawn_rate = 200 # chance-based
def spawn_pickup():
x = random.randint(220, WIDTH - 220)
y = -30
pickups.append([x, y])
def update_pickups(pickups):
for pickup in pickups[:]:
pickup[1] += 3
if pickup[1] > HEIGHT:
pickups.remove(pickup)
return pickups
Integrate and test that pickups appear intermittently and move down the screen.
Step-6: Collision Detection and Scoring
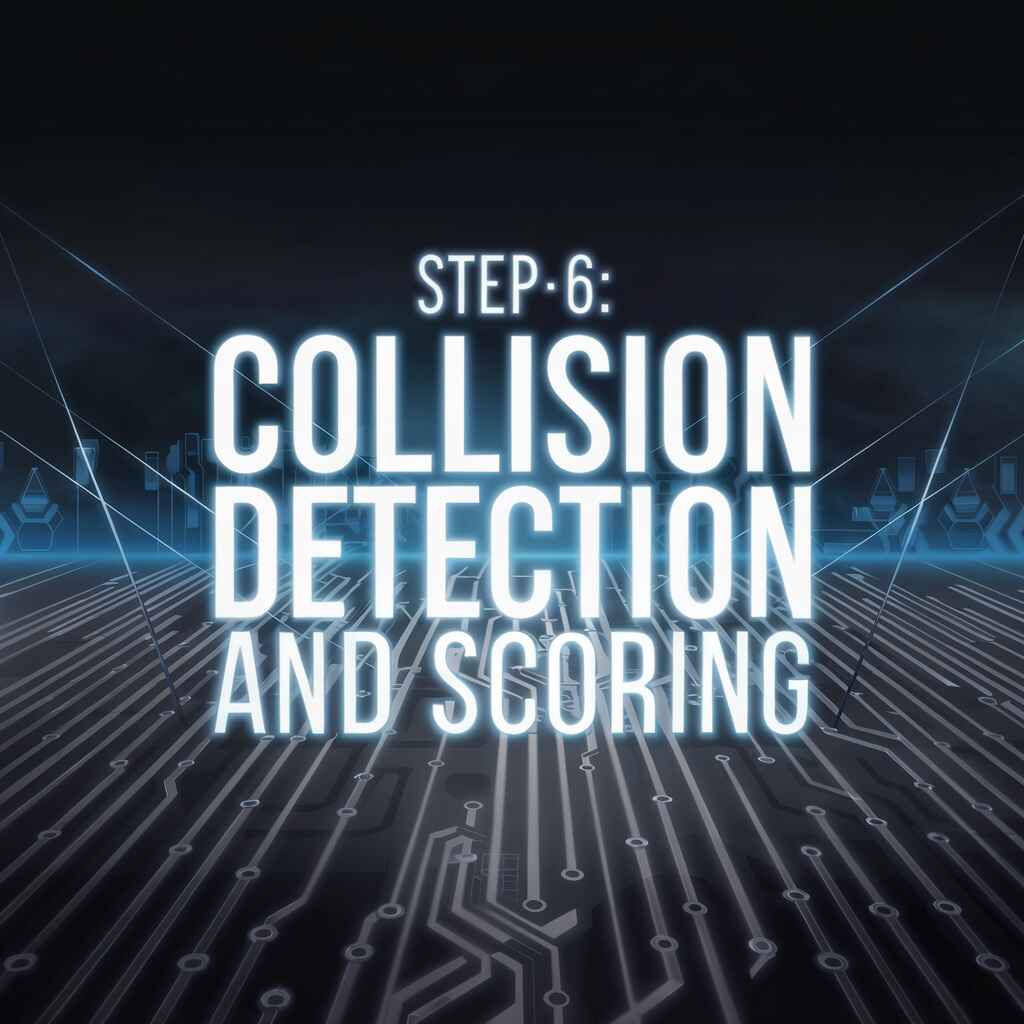
The game must detect when the player collides with an obstacle or collects a pickup. This section also updates the score.
def check_collision(rect1, rect2):
return rect1.colliderect(rect2)
score = 0
def update_score(current_score):
return current_score + 1 # Increase score over time
Inside your game loop, create rectangles for the player, obstacles, and pickups using pygame.Rect, and check for collisions accordingly. For obstacles, end the game on collision; for pickups, increase the score and remove the pickup.
Integrating All Modules into the Final Game
Now that each piece works individually, it’s time to put everything together into a single, coherent game. This is your complete python games code for Neon Racer.
Below is the integrated end-to-end version:
Complete Code: Neon Racer
import pygame, random, sys
# Initialize Pygame
pygame.init()
# Screen dimensions and setup
WIDTH, HEIGHT = 800, 600
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Neon Racer")
clock = pygame.time.Clock()
# Colors and fonts
BG_COLOR = (20, 20, 30)
PLAYER_COLOR = (0, 255, 255) # Neon cyan for the player
OBSTACLE_COLOR = (255, 50, 50)
PICKUP_COLOR = (50, 255, 50)
ROAD_COLOR = (50, 50, 50)
font = pygame.font.Font(None, 36)
# Game Variables
player_x = WIDTH // 2
player_y = HEIGHT - 120
player_speed = 7
obstacles = []
pickups = []
frame_count = 0
score = 0
# Road variables
road_y = 0
road_speed = 5
obstacle_spawn_rate = 30 # frames
# Main Game Loop
running = True
while running:
screen.fill(BG_COLOR)
# Draw scrolling road (two sections for continuous scroll)
road_y += road_speed
if road_y >= HEIGHT:
road_y = 0
pygame.draw.rect(screen, ROAD_COLOR, (200, road_y, 400, HEIGHT))
pygame.draw.rect(screen, ROAD_COLOR, (200, road_y - HEIGHT, 400, HEIGHT))
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# Player movement
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT] and player_x > 200:
player_x -= player_speed
if keys[pygame.K_RIGHT] and player_x < 540: # 800 - 260 to keep within road
player_x += player_speed
# Spawn obstacles
frame_count += 1
if frame_count % obstacle_spawn_rate == 0:
x = random.randint(220, 580)
obstacles.append([x, -50, random.randint(4, 7)])
# Spawn pickups occasionally
if random.randint(1, 200) == 1:
x = random.randint(220, 580)
pickups.append([x, -30])
# Update obstacles and draw them
for obstacle in obstacles[:]:
obstacle[1] += obstacle[2]
obs_rect = pygame.Rect(obstacle[0], obstacle[1], 40, 40)
pygame.draw.rect(screen, OBSTACLE_COLOR, obs_rect)
if obstacle[1] > HEIGHT:
obstacles.remove(obstacle)
# Update pickups and draw them
for pickup in pickups[:]:
pickup[1] += 3
pick_rect = pygame.Rect(pickup[0]-15, pickup[1]-15, 30, 30)
pygame.draw.ellipse(screen, PICKUP_COLOR, pick_rect)
if pickup[1] > HEIGHT:
pickups.remove(pickup)
# Create player rectangle and draw
player_rect = pygame.Rect(player_x, player_y, 60, 30)
pygame.draw.rect(screen, PLAYER_COLOR, player_rect)
# Collision detection for obstacles
for obstacle in obstacles:
obs_rect = pygame.Rect(obstacle[0], obstacle[1], 40, 40)
if player_rect.colliderect(obs_rect):
print("Game Over!")
running = False
# Collision detection for pickups
for pickup in pickups[:]:
pick_rect = pygame.Rect(pickup[0]-15, pickup[1]-15, 30, 30)
if player_rect.colliderect(pick_rect):
score += 100 # Bonus for collecting a pickup
pickups.remove(pickup)
# Update score over time
score += 1
score_text = font.render(f"Score: {score}", True, (255, 255, 255))
screen.blit(score_text, (10, 10))
pygame.display.update()
clock.tick(60)
pygame.quit()
sys.exit()
Test Your DSA Skills: Python DSA Quiz Part-01
Any Questions on Our Python Game Code?
In this guide, you learned to build Neon Racer, a unique game developed with python game code using Pygame. We covered everything from setting up your environment, creating a scrolling background, and handling player movement, to generating obstacles, pickups, collision detection, and scoring. Each section is a puzzle piece that you combine to form the complete game.
This fresh, step-by-step guide on python codes for games provides a solid foundation for further enhancements—such as adding sound, a game over screen, or multiple levels—to make your game even more engaging. Enjoy coding Neon Racer, and feel free to experiment and add your own creative twists!
Feel free to ask if you need further clarifications or additional features. Don’t miss to subscribe to our YouTube channel for more such engaging updates.