Python provides multiple ways to increment and decrement values, which are commonly used for:
✅ Controlling iteration within loops
✅ Managing counters in simulations or data processing
✅ Adjusting values dynamically in mathematical computations
Unlike languages like C or Java, Python does not support x++ or x– operators. Instead, it encourages an explicit and readable approach using += for incrementing and -= for decrementing values.
This guide covers basic methods, advanced techniques, common errors, and best practices for incrementing and decrementing values in Python.
Why Doesn’t Python Have x++ and x–?
In most languages that support ++
and --
, these operators can function in two ways:
✔ Pre-increment (++x
): Increases the value before using it in an expression.
✔ Post-increment (x++
): Uses the current value in an expression before increasing it.
Python intentionally omits these operators for the following reasons:
1️⃣ Clarity and Readability: Python follows the principle of explicit over implicit, meaning all operations should be clearly defined. Since pre- and post-increment operators often introduce subtle side effects in expressions, Python enforces explicit incrementing.
2️⃣ Avoids Ambiguity in Expressions: In languages like C++, x = y++ + ++y;
can yield unexpected behaviour. Python eliminates this confusion by requiring explicit assignments:
x = 5
x += 1 # Explicitly increases x by 1
3️⃣ Immutable Integer Objects: Python integers are immutable, meaning every arithmetic operation results in a new integer object rather than modifying the existing one. Implementing x++
or x--
would not align with Python’s design.
To maintain consistency, simplicity, and predictable behaviour, Python enforces explicit syntax using +=
and -=
operators.
How to Increment a Value in Python
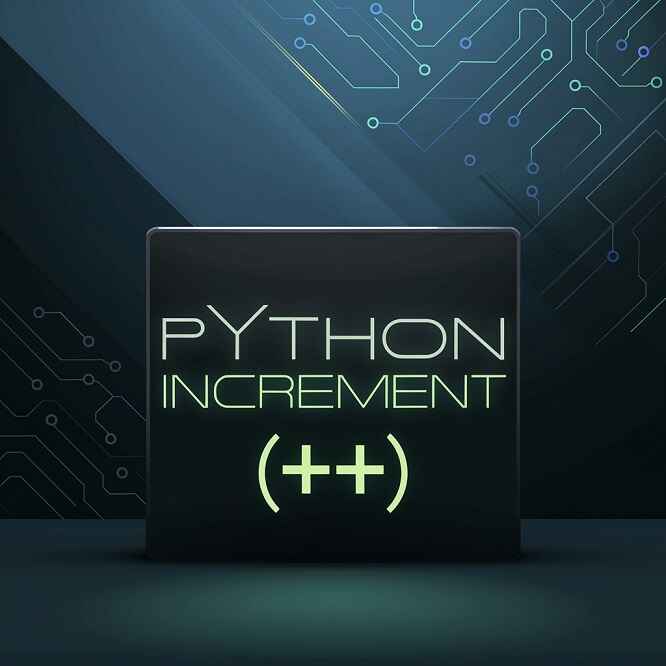
Since x++
is not a valid operation in Python, the correct way to increase a value is by using the compound assignment operator +=
.
Incrementing by 1
x = 5
x += 1 # Equivalent to x = x + 1
print(x) # Output: 6
This operation takes the existing value of x
, adds 1
, and stores the result back into x
.
Incrementing by a Custom Step
For cases where a variable needs to be increased by a value greater than 1
, the step size can be adjusted:
x = 10
x += 5 # Increases x by 5
print(x) # Output: 15
Incrementing in Loops
Incrementing is frequently used within iterative structures such as while
loops:
x = 0
while x < 5:
print(x) # Outputs: 0, 1, 2, 3, 4
x += 1 # Increments x by 1
Each loop iteration updates x
explicitly, ensuring it progresses toward the exit condition.
How to Decrement a Value in Python
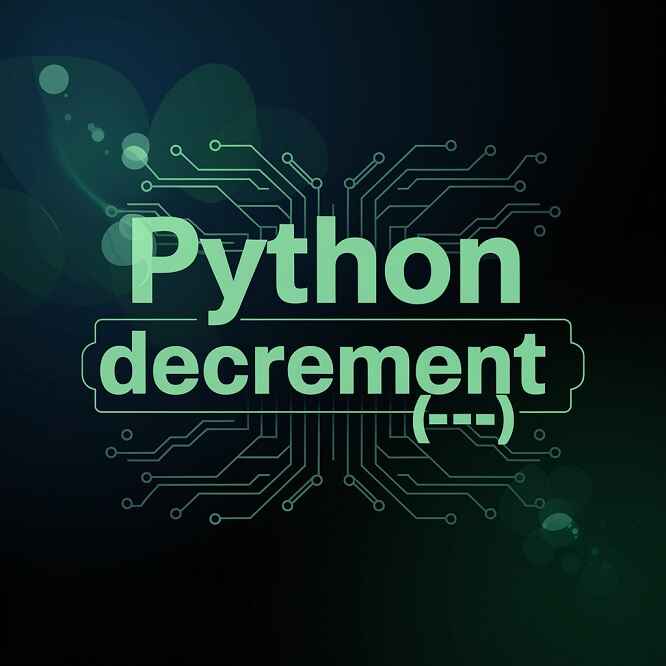
Python does not support x--
, but a value can be reduced using the -=
operator.
Decreasing by 1
x = 10
x -= 1 # Equivalent to x = x - 1
print(x) # Output: 9
Decreasing by a Custom Step
Similar to incrementing, decrementing can be applied using a step value:
x = 20
x -= 5 # Decreases x by 5
print(x) # Output: 15
Decrementing in Loops
Decrementing is often required when iterating in reverse order:
x = 5
while x > 0:
print(x) # Outputs: 5, 4, 3, 2, 1
x -= 1
This is useful for countdown sequences, reversing iterations, or processing stacks of data.
Advanced Increment and Decrement Techniques
Using range()
for Stepwise Iteration
Instead of manually incrementing or decrementing within loops, Python’s range()
function provides efficient numeric iteration.
Incrementing with range()
for i in range(0, 10, 2): # Starts at 0, increments by 2
print(i) # Output: 0, 2, 4, 6, 8
Decrementing with range()
for i in range(10, 0, -2): # Starts at 10, decrements by 2
print(i) # Output: 10, 8, 6, 4, 2
Implementing Custom Increment and Decrement Functions
Encapsulating arithmetic operations within functions enhances modularity and reusability:
def increment(value, step=1):
return value + step
print(increment(10)) # Output: 11
print(increment(10, 5)) # Output: 15
def decrement(value, step=1):
return value - step
print(decrement(10)) # Output: 9
print(decrement(10, 3)) # Output: 7
Common Mistakes and Debugging Tips
Incorrect Usage of x++
or x--
x = 5
x++ # SyntaxError: invalid syntax
Correction:
Use x += 1
or x -= 1
instead.
Forgetting to Update the Counter in Loops
x = 5
while x > 0:
print(x) # Infinite loop! (x never decreases)
Correction:
Ensure the counter is updated inside the loop.
while x > 0:
print(x)
x -= 1 # Ensures loop terminates
Using +=
or -=
on Incompatible Data Types
text = "hello"
text += 1 # TypeError: can only concatenate str (not "int") to str
Correction:
Ensure that +=
and -=
are used with numeric types or compatible data structures.
Any Questions on Python Increment or Decrement Operators?
This guide has provided a thorough overview of incrementing and decrementing values in Python, explaining:
✅ Why x++
and x--
are not supported
✅ The correct usage of +=
and -=
for modifying values
✅ Advanced techniques using range()
, loops, and functions
✅ Common mistakes and debugging strategies
Check the summary from the below table:
Operation | Python Equivalent | Example |
---|---|---|
Increment by 1 | x += 1 | x = 5;x += 1 → 6 |
Decrement by 1 | x -= 1 | x = 5; x -= 1 → 4 |
Increment by N | x += N | x = 5; x += 3 → 8 |
Decrement by N | x -= N | x = 5; x -= 3 → 2 |
Next Steps
💡 Try these techniques in real-world applications
📢 Share this guide with other Python learners
📩 Explore more Python tutorials to expand your knowledge
🔔 Click here to subscribe our YouTube channel!
🔥 Happy coding! 🚀