Need a factorial program in Python? This guide covers simple and advanced ways to calculate factorials using loops, recursion, and optimized methods. Find out which approach works best for you – and don’t miss the benchmark graph comparing their performance.
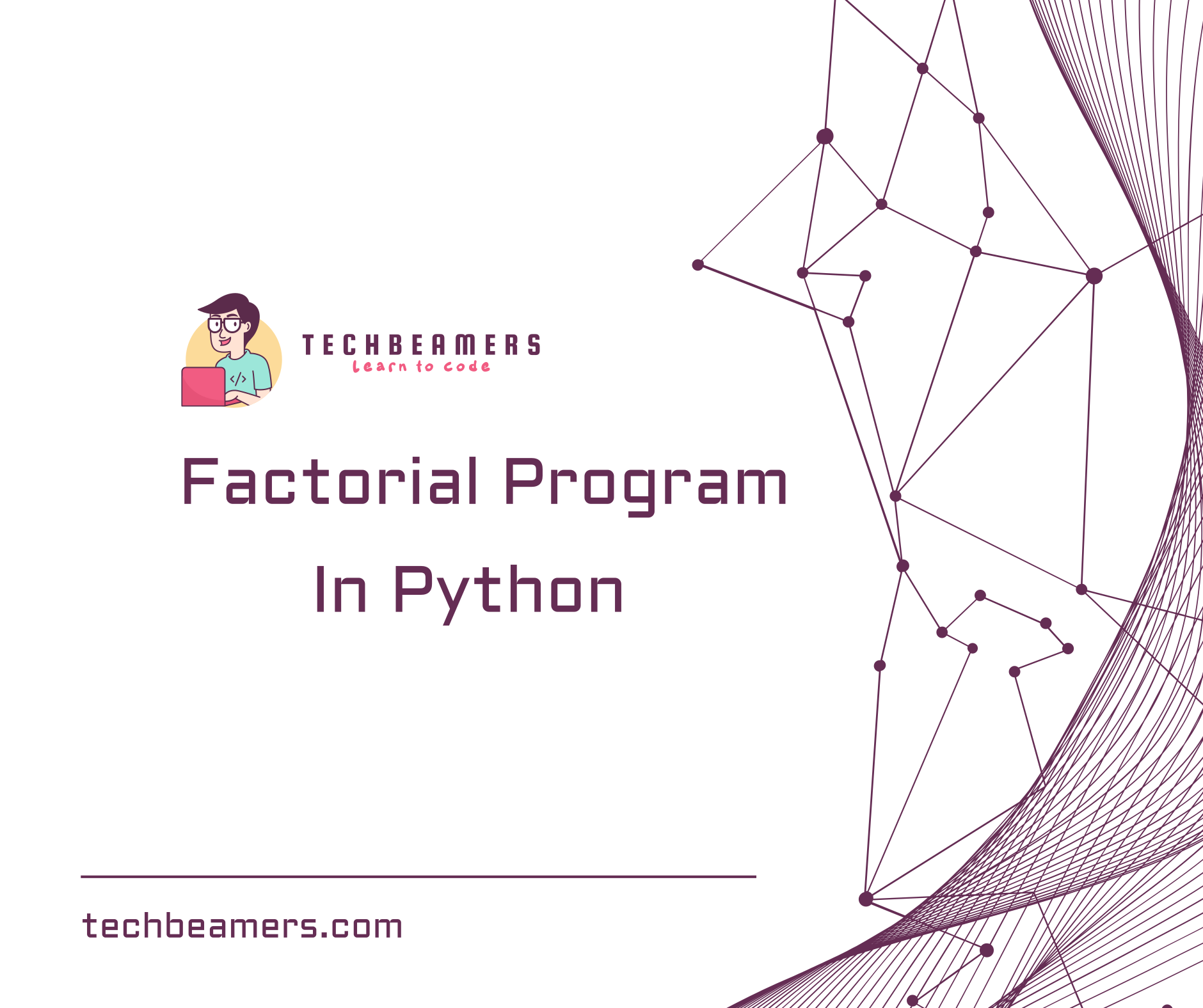
Simple Factorial Program in Python
Here’s the easiest way to calculate factorial in Python:
import math
def get_positive_integer():
"""
This function repeatedly prompts the user for a valid non-negative integer.
It ensures that only a valid input is accepted before proceeding.
"""
while True:
try:
num = int(input("Enter a non-negative integer: "))
if num < 0:
print("Factorial is only defined for non-negative integers. Try again.")
else:
return num
except ValueError:
print("Invalid input. Please enter a valid integer.")
num = get_positive_integer()
print("Factorial of", num, "is", math.factorial(num))
Python’s built-in math.factorial() function is the most efficient way to compute factorials. Now, let’s explore alternative methods.
📌 Note: The get_positive_integer() function is used in all examples below to ensure valid user input. You might have noticed the multiline comment inside the function explaining its purpose – if you want to learn more about writing such comments in Python, check out our guide on writing multiline comments in Python.
Factorial Program in Python – Different Methods
Below are various ways to write a factorial program in Python, suitable for different scenarios.
1️⃣ Using a Loop (Iterative Method)
def factorial_iterative(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
num = get_positive_integer()
print("Factorial of", num, "is", factorial_iterative(num))
Why use it? Simple and easy to understand. Good for beginners.
2️⃣ Using Recursion
def factorial_recursive(n):
if n == 0 or n == 1:
return 1
return n * factorial_recursive(n - 1)
num = get_positive_integer()
print("Factorial of", num, "is", factorial_recursive(num))
Why use it? Useful for understanding recursion but not ideal for large numbers due to stack overflow risk.
3️⃣ Using Functional Programming (reduce())
from functools import reduce
def factorial_reduce(n):
return reduce(lambda x, y: x * y, range(1, n + 1), 1)
num = get_positive_integer()
print("Factorial of", num, "using reduce is", factorial_reduce(num))
Why use it? A compact approach using Python’s functional programming tools.
4️⃣ Using NumPy for Vectorized Computation
import numpy as np
def factorial_numpy(n):
return np.prod(np.arange(1, n + 1))
num = get_positive_integer()
print("Factorial of", num, "using NumPy is", factorial_numpy(num))
Why use it? Faster for large computations by leveraging NumPy’s array operations.
5️⃣ Using Memoization (Optimized Recursion with Caching)
from functools import lru_cache
@lru_cache(maxsize=None)
def factorial_memo(n):
if n == 0 or n == 1:
return 1
return n * factorial_memo(n - 1)
num = get_positive_integer()
print("Factorial of", num, "using memoization is", factorial_memo(num))
Why use it? Optimizes recursion by storing previous results, making repeated calls faster.
6️⃣ Parallel Computing for Large Factorials (Multiprocessing)
from multiprocessing import Pool
def factorial_partial(start_end):
start, end = start_end
result = 1
for i in range(start, end + 1):
result *= i
return result
def factorial_parallel(n):
num_workers = 4
chunk_size = n // num_workers
ranges = [(i * chunk_size + 1, (i + 1) * chunk_size) for i in range(num_workers)]
with Pool(processes=num_workers) as pool:
results = pool.map(factorial_partial, ranges)
final_result = 1
for r in results:
final_result *= r
return final_result
num = get_positive_integer()
print("Factorial of", num, "using multiprocessing is", factorial_parallel(num))
Why use it? Best for handling extremely large numbers using multiple CPU cores.
7️⃣ Factorial Using Lambda Functions (One-Liner Approach)
factorial_lambda = lambda n: 1 if n == 0 else n * factorial_lambda(n - 1)
num = get_positive_integer()
print("Factorial of", num, "using lambda is", factorial_lambda(num))
Why use it? A quick and compact way to compute factorial using a lambda function.
Run a Benchmark Test on These Factorial Methods! 🚀
To understand which factorial method performs best, we ran a benchmark comparing 7 different implementations. Want to test them on your own system? Get the benchmark script below.
🔽 Download the Python Benchmark Script (ZIP File) → factorial_benchmark.py
This script runs comprehensive benchmark tests, measuring the execution time of all 7 factorial methods across different values of n. The results show:
✅ Let’s analyse the observations from the above benchmark graph.
1️⃣ Memoization (Green Squares) is the Best (Fastest Execution Time)
- Stays near zero execution time across all values of n.
- Caching speeds up computation significantly.
2️⃣ NumPy (Brown Line) is the Second Best
- Consistently performs well due to vectorized computation.
3️⃣ Recursion (Orange Line) is the Worst
- Execution time increases steeply as
n
grows. - Struggles due to stack overhead & function call depth.
4️⃣ Loop & While Loop Perform Decently
- Better than recursion but slower than memoization & NumPy.
5️⃣ Python’s Built-in math.factorial()
(Red Triangles) is Highly Optimized
- Fastest among non-cached methods, but slightly behind memoization.
Frequently Asked Questions (FAQ)
❓ What is the most efficient way to compute factorial in Python?
For small numbers, math.factorial(n) is the best choice. For large numbers, multiprocessing or NumPy performs better.
❓ Why does recursion fail for very large numbers?
Recursion can cause a stack overflow when n is too large. If you need recursion, lru_cache can help, but an iterative approach is safer.
❓ Can I compute the factorial of a decimal or negative number?
No, factorial is only defined for non-negative integers. If you need fractional factorials, look into the Gamma function.
❓ What’s the best method for coding interviews or exams?
For quick implementations, math.factorial(n) is best. If recursion is expected, use factorial_recursive(n), but be mindful of limits.
❓ When should I use multiprocessing for factorials?
For extremely large numbers (e.g., 5000!), multiprocessing can speed up calculations by using multiple CPU cores.
Final Thoughts on Factorial Programs in Python
A factorial program in Python can be implemented in many ways, from simple loops to advanced multiprocessing. Depending on your needs, you can choose an approach that balances readability, performance, and scalability.
🚀 Next Steps: Try different methods, measure performance, and see which one works best for your use case!
💡 Did you find this useful? Share your thoughts and let us know how you use factorial in your projects! Also, subscribe to our YouTube channel for the latest Python tutorials and coding insights.