Cross-browser testing is essential in web development. It ensures your app works across different browsers. Browsers can render websites differently, causing issues in appearance and behaviour. Playwright is a modern tool that solves this problem. Developed by Microsoft, it offers a reliable solution for cross-browser testing. Playwright lets developers and testers automate tests on Chrome, Firefox, and Edge easily.
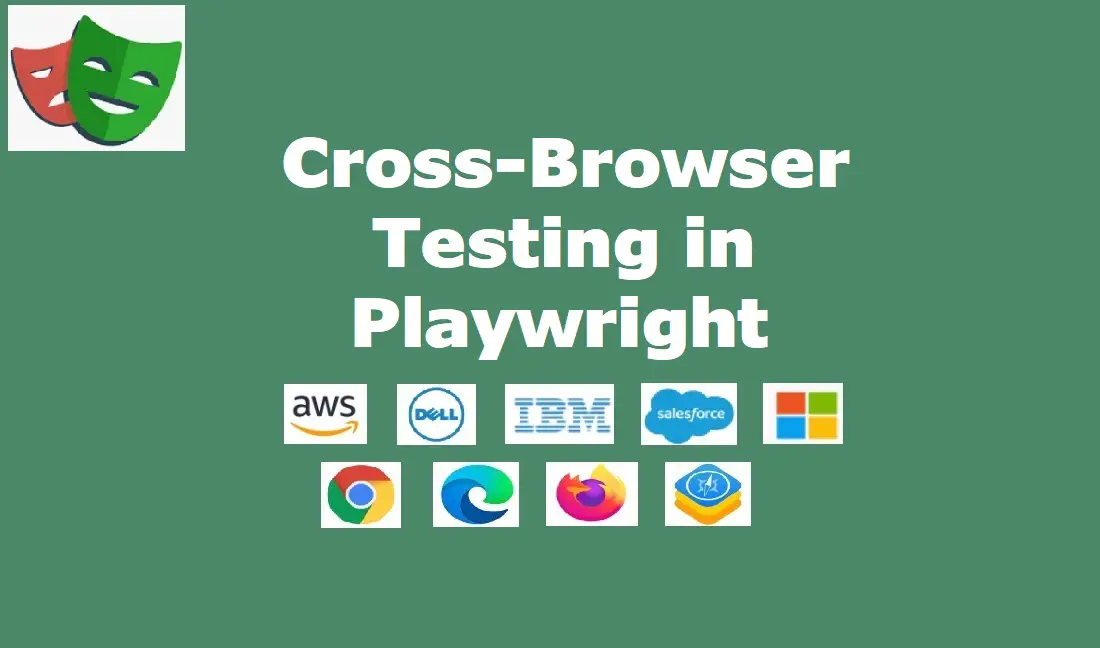
You make like to know: What is Playwright Testing?
How does Playwright Handle Cross-browser Testing?
In this guide, we’ll explore how Playwright handles cross-browser testing, why it’s a powerful tool for this purpose, and how to get started with it.
What is Cross-Browser Testing?
Cross-browser testing involves verifying that a website or web application performs as expected on different browsers and platforms. This is crucial because:
- Rendering Across Browsers: The same web page can look different on various browsers.
- JavaScript Compatibility: Browsers handle the latest JavaScript features in different ways.
- Performance Differences: Each browser has its own performance, impacting page load speed and user interaction smoothness.
In the past, cross-browser testing was a complex and time-consuming task, often requiring different tools and scripts for each browser. However, Playwright simplifies the process by offering a unified solution for testing multiple browsers at once.
How Playwright Handles Cross-Browser Testing?
Support for Major Browsers:
Playwright supports the three major browser engines:
- Chromium (used by Chrome and Edge)
- Firefox
- WebKit (used by Safari)
With Playwright, you can run tests across all these browsers without needing to install each browser separately. The same test scripts can be used for all browsers, making it easier to maintain tests and ensure consistency across platforms.
Unified API for All Browsers:
One of Playwright’s strongest points is its unified API. Unlike other tools where each browser may require slightly different setups or code to interact with it, Playwright abstracts the differences between browsers. Whether you’re working with Chromium, Firefox, or WebKit, the Playwright API remains consistent. This means you don’t need to worry about browser-specific variations in your test scripts.
For example, you can launch and interact with any of the three supported browsers using the same code:
const { chromium, firefox, webkit } = require('playwright');
// Launch browsers
const chromiumBrowser = await chromium.launch();
const firefoxBrowser = await firefox.launch();
const webkitBrowser = await webkit.launch();
Real-World Browser Interaction:
Playwright goes beyond simulating browsers; it runs actual browser instances. This makes your tests more accurate, as they reflect how users will interact with your app. You can test in headless mode (where the browser runs in the background) or headed mode (with a visible UI). It gives you flexibility to choose the best setup for your testing needs.
Automatic Management of Browser-Specific Features:
Playwright automatically handles browser-specific quirks for you. For example:
- It takes care of rendering differences between browsers.
- Features unique to each browser, like service workers and storage mechanisms, are managed through Playwright’s API, so you don’t have to deal with the technical details.
- Playwright also ensures consistent JavaScript execution across all browsers, which is often a challenge in cross-browser testing.
Parallel Testing Across Browsers:
Playwright helps you to run tests in parallel. You can set up multiple browsers. This means you can run the test suite on Chrome, Firefox, and Safari concurrently. This significantly speeds up your test execution.
Here’s an example of running tests in parallel on multiple browsers:
const { chromium, firefox, webkit } = require('playwright');
const browsers = [chromium, firefox, webkit];
for (let browserType of browsers) {
const browser = await browserType.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
console.log(await page.title()); // Print page title for each browser
await browser.close();
}
Go deeper into coding, check out: Playwright Python Tutorial
Network Interception and Conditions:
Playwright allows you to intercept network requests and responses, so you can simulate different network conditions (such as slow networks, offline mode, etc.) for each browser. This helps ensure that your app works well under a variety of conditions.
Headless Browsers for CI/CD:
Playwright is perfect for Continuous Integration (CI) and Continuous Delivery (CD) environments because of its support for headless browsers. Running Playwright tests in headless mode (where browsers don’t display their UI) speeds up the testing process and makes it easier to integrate with tools like Jenkins, GitHub Actions, and GitLab CI.
Why Cross-Browser Testing Matters with Playwright?
- Consistency Across Browsers:
Playwright ensures your app works the same across different browsers. If a bug appears in one browser, Playwright helps you check if it happens in others, ensuring consistency everywhere. - Faster Feedback for Developers:
As Playwright can run tests in parallel on multiple browsers, developers get quick feedback. This helps them be sure their updates won’t break the app in browsers they haven’t explicitly tested. - Better User Experience:
Since Playwright tests in real browsers, it’s easier to catch subtle issues that might go unnoticed. Because of this, the user experience is smooth across all platforms - Support for Older Browsers:
With Playwright’s WebKit support, you can test on Safari, including older versions like Safari 11 or earlier versions of Chrome/Edge, making it easier to maintain compatibility with legacy browsers.
How to Perform Cross-Browser Testing with Playwright?
Let’s go over a basic example of how you can perform cross-browser testing with Playwright.
Install Playwright: First, you need to install Playwright:
$ npm init -y
$ npm install playwright
Write a Cross-Browser Test: Here’s a simple script to test a webpage across Chrome, Firefox, and Safari (WebKit):
const { chromium, firefox, webkit } = require('playwright');
async function runTests() {
const browsers = [chromium, firefox, webkit];
for (let browserType of browsers) {
const browser = await browserType.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
console.log(`Testing on ${browserType.name()}: ${await page.title()}`);
await browser.close();
}
}
runTests();
Run the Test: To run your tests, use the following command:
$ node cross-browser-test.js
Playwright will automatically launch the three browsers, navigate to the page, and print the title of the page for each browser.
Conclusion
Playwright is great for cross-browser testing. It supports major browsers like Chrome, Firefox, and Safari (WebKit) through one API. Its speed, reliability, and ability to handle browser differences make it perfect for developers. It helps ensure applications work well across different platforms.
With Playwright, you can automate tests with little setup. You can also run tests in parallel, saving time. This leads to a smoother user experience. Whether you’re testing modern web apps or older browsers, Playwright makes cross-browser testing easy and efficient.
If you found this useful, subscribe to our YouTube channel for more tips and insights.