When explaining the Playwright framework in an interview, you want to structure your answer to cover its core features, advantages over Selenium, architecture, and practical use cases while keeping it concise and clear. Here’s how you can approach it:
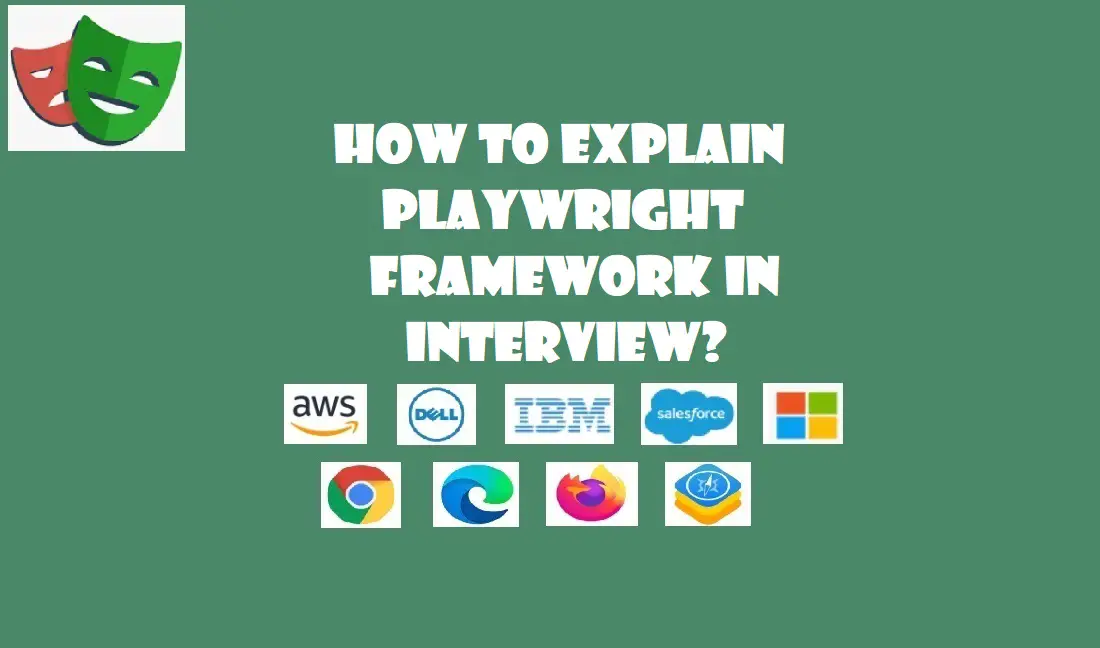
Explain Playwright Framework in Interview
Let’s understand the best possible way to explain the playwright framework when asked in interviews.
Introduction to Playwright
Playwright is an open-source end-to-end testing framework developed by Microsoft that enables fast, reliable, and cross-browser automation for web applications. It supports multiple browsers (Chromium, Firefox, WebKit) and various programming languages (JavaScript, TypeScript, Python, C#, and Java).
Example response:
Playwright is a modern web automation framework that supports cross-browser testing, parallel execution, and powerful API testing capabilities. It’s designed to overcome Selenium’s limitations, offering better speed, reliability, and ease of debugging.
How Playwright Works (Architecture Overview)
Playwright works by automating real browsers using its headless or headed mode, ensuring reliable execution. Unlike Selenium, which relies on WebDriver, Playwright directly communicates with the browser using the DevTools Protocol, reducing execution overhead.
Key components of Playwright:
- BrowserContext – Enables testing in an isolated session.
- Page – Represents a single tab in a browser.
- Selectors & Locators – Auto-waiting for elements to be ready before interacting.
- Assertions & Auto-Waiting – Built-in test expectations for stable automation.
Example response:
Playwright operates by launching browsers in an isolated context and interacting with them using powerful APIs that handle auto-waiting, network interception, and real-time debugging. This direct communication makes it faster and more reliable than Selenium.
Why Choose Playwright Over Selenium?
Feature | Playwright | Selenium |
---|---|---|
Speed | Faster (direct browser control) | Slower (uses WebDriver) |
Parallel Execution | Built-in support | Needs Grid setup |
Auto-Waiting | Yes | Manual handling |
Browser Support | Chromium, Firefox, WebKit | More extensive but less efficient |
API Testing | Yes, built-in | No |
Network Interception | Yes, advanced capabilities | Limited |
Test Generator | Built-in code recorder | No built-in recorder |
Example response:
Compared to Selenium, Playwright is faster due to direct browser interaction, supports parallel execution natively, and includes built-in features like network mocking, API testing, and headless execution, making it a more modern automation tool.
Core Features of Playwright
- Cross-Browser Testing – Supports Chrome, Firefox, and Safari.
- Headless Mode & Parallel Execution – Runs multiple tests at once, improving speed.
- Network Interception & Mocking – Allows testing offline behavior and simulating API responses.
- Built-in Assertions – Includes powerful assertion APIs for better reliability.
- Visual Testing – Screenshots, videos, and trace recording for debugging.
Playwright JavaScript Example
If asked to demonstrate Playwright, here’s a basic test script in JavaScript:
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch({ headless: false });
const page = await browser.newPage();
await page.goto('https://example.com');
await page.fill('#username', 'testUser');
await page.fill('#password', 'password123');
await page.click('button[type="submit"]');
await page.waitForSelector('#dashboard');
console.log("Login successful!");
await browser.close();
})();
Example response:
Here’s a simple Playwright test that launches a browser, logs into a website, and verifies the dashboard loads. It handles automatic waiting and retries, making automation more reliable.
Playwright Python Example
You can also explain with a Playwright Python example. Here’s a basic test script in Python:
from playwright.sync_api import sync_playwright
def test_login():
with sync_playwright() as p:
# Launch the browser (headed mode for debugging)
browser = p.chromium.launch(headless=False)
page = browser.new_page()
# Navigate to the login page
page.goto("<Specify a web page url>")
# Fill in login credentials
page.fill("#username", "testUser")
page.fill("#password", "testPassword")
# Click login button
page.click("button[type='submit']")
# Wait for dashboard to load
page.wait_for_selector("#dashboard")
# Print success message
print("Login successful!")
# Close browser
browser.close()
if __name__ == "__main__":
test_login()
Key Features in This Code:
✅ sync_playwright – Runs Playwright in sync mode for simplicity.
✅ browser.new_page() – Opens a new browser tab.
✅ goto() – Navigates to the webpage.
✅ fill() – Enters username and password.
✅ click() – Clicks the login button.
✅ wait_for_selector() – Waits for the dashboard element to confirm login success.
✅ headless=False – Runs in visible mode for debugging.
When to Use Playwright?
- UI Automation Testing – End-to-end testing of web applications.
- API Testing – Fetch and validate API responses.
- Web Scraping – Extract data from dynamic web pages.
- Performance Testing – Simulate real user interactions.
- CI/CD Pipelines – Integrates with Jenkins, GitHub Actions, and Azure DevOps.
Example response:
Playwright is ideal for end-to-end testing, API testing, and performance testing. Since it integrates well with CI/CD pipelines, it ensures robust and scalable test automation.
Common Playwright Interview Questions
- What are the advantages of Playwright over Selenium?
- How does Playwright handle network requests?
- Explain the difference between browser.newContext() and browser.newPage().
- How does Playwright support parallel execution?
- Can Playwright be used for API testing?
- What are Playwright fixtures, and how do they help in testing?
Example response:
One common question is how Playwright manages test isolation. Playwright uses BrowserContext to create independent sessions, ensuring tests don’t interfere with each other, unlike Selenium, where a new browser instance is needed for isolation.
Summary: Explaining the Playwright Framework
When explaining Playwright in an interview: ✔ Keep it structured – Define what Playwright is, how it works, and why it’s better than Selenium.
✔ Show practical usage – Explain how it integrates with testing strategies.
✔ Provide a simple code example – This shows your hands-on experience.
✔ Be ready for follow-up questions – Understand parallel execution, network mocking, and Playwright’s unique features.
Would you like help preparing for any specific Playwright interview questions? 😊 Also, don’t forget to subscribe to our YouTube channel for more tutorials and tips! 🚀🎥