Explore 20 most important Playwright interview questions and answers on basic, advanced, and scenario-based topics for your next automation testing interview. Playwright is faster than Selenium. It is widely used by major companies like Amazon, Dell, IBM, Salesforce, and Microsoft. It supports all major browsers, including Chrome, Safari, Firefox, and Edge, along with browser engines like WebKit.
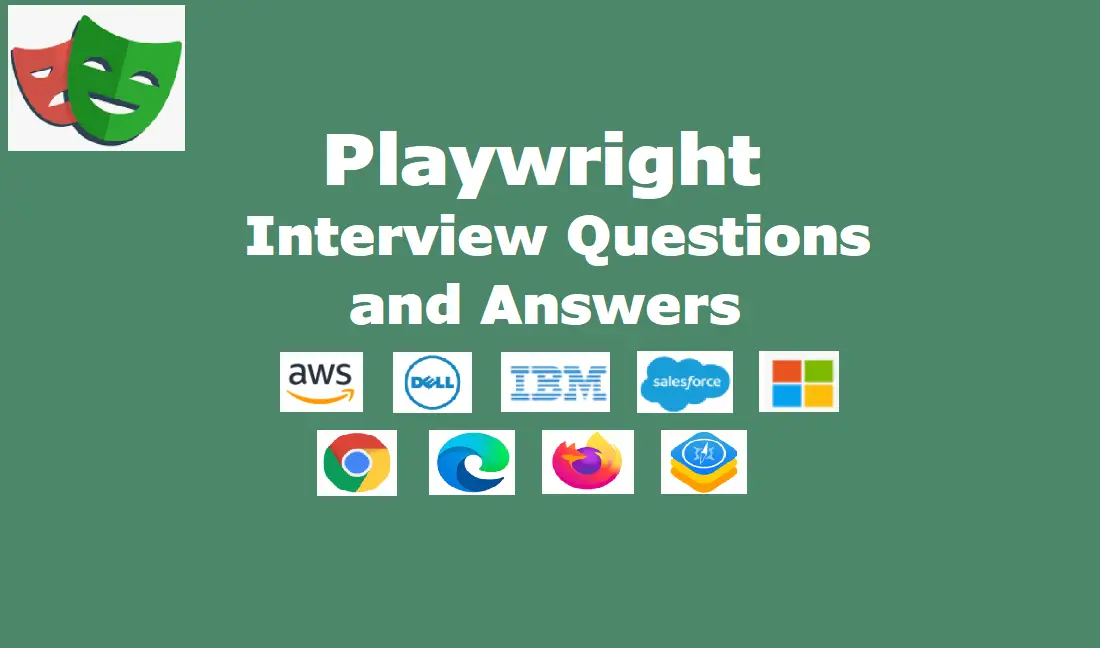
You may also like: Web Scraping using Selenium Python
Basic Playwright interview questions
1. What is Playwright?
Answer: Playwright is an open-source automation framework for web testing. It supports multiple browsers (Chromium, Firefox, WebKit) and allows automation in various languages like Python, Java, and JavaScript.
- It provides auto-waiting, meaning no need for explicit waits.
- It supports headless mode for faster execution.
- It enables network interception & mocking.
- Playwright is faster than Selenium due to better architecture.
Example:
from playwright.sync_api import sync_playwright
with sync_playwright() as p:
browser = p.chromium.launch()
page = browser.new_page()
page.goto("<specify website url here>")
print(page.title())
browser.close()
2. How do you install and use Playwright in Python?
Answer: We can use Python’s pip installer to install the Playwright package for both Windows and Linux:
pip install playwright
playwright install
Basic usage:
from playwright.sync_api import sync_playwright
with sync_playwright() as p:
browser = p.chromium.launch()
page = browser.new_page()
page.goto("enter website url here")
print(page.title())
browser.close()
3. What is page in Playwright?
Answer: A page in Playwright represents a browser tab where we can navigate, interact with elements, and perform various testing actions. It is created using browser.new_page().
Example:
page = browser.new_page()
page.goto("<specify a website url>")
print(page.title())
4. How do you handle elements in Playwright?
Answer: With Playwright, we handle web elements in the following two steps:
- Use locator() to find elements.
- Use click(), fill(), type(), etc., for interactions.
Example:
page.fill("#username", "testuser")
page.click("#login-button")
5. How do you handle dropdowns in Playwright?
Answer: In Playwright, we can handle dropdowns using the page class and the select_option() method. This method allows selecting options based on value, label, or index.
# Select by value
page.select_option("#dropdown", "option1")
# Select by label
page.select_option("#dropdown", label="Option 1")
# Select by index
page.select_option("#dropdown", index=2)
# Select multiple options (if supported)
page.select_option("#dropdown", ["option1", "option2"])
6. How do you handle alerts/popups in Playwright?
Answer: In Playwright, we can handle JavaScript alerts, confirmations, and prompts using the page class and the on(“dialog”) event listener. The Dialog object provides methods like accept() to confirm and dismiss() to cancel the alert.
Example:
Handling an alert and accepting it:
def handle_alert(dialog):
print("Alert text:", dialog.message)
# Clicks OK on the alert
dialog.accept()
page.on("dialog", handle_alert)
Dismissing an alert:
# Clicks Cancel
page.on("dialog", lambda dialog: dialog.dismiss())
Handling a prompt and providing input:
def handle_prompt(dialog):
print("Prompt text:", dialog.message)
# Enters "Test input" and clicks OK
dialog.accept("Test input")
page.on("dialog", handle_prompt)
This approach ensures that Playwright automatically listens for and handles popups when they appear.
Advanced Playwright interview questions
7. How do you take a screenshot in Playwright?
Answer: In Playwright, we can capture screenshots using the screenshot() method provided by the page class. Screenshots help with debugging, visual testing, and UI verification.
The code to take a basic screenshot:
page.screenshot(path="screenshot.png")
For capturing a full-page screenshot:
page.screenshot(path="full.png", full_page=True)
The code to take a screenshot of a specific element:
page.locator("#element-id").screenshot(path="element.png")
And, finally, we can also add some delay while taking screenshots.
import time
# Wait before taking a screenshot
time.sleep(2)
page.screenshot(path="delayed.png")
This ensures flexibility in capturing web page states efficiently.
8. How do you handle iframes in Playwright?
Answer: In Playwright, we can interact with iframes using the frame() or frame_locator() methods. Iframes are embedded HTML documents, and to interact with elements inside them, we must first locate the iframe and then perform actions within its context.
# Locate by name
iframe = page.frame(name="iframe_name")
# Locate by URL
iframe = page.frame(url="<specify the base url>/iframe")
If the iframe loads dynamically, we should wait for it before performing actions.
iframe = page.wait_for_selector("iframe")
frame = iframe.content_frame()
frame.fill("#input", "Playwright")
Once loaded, we should access the frame locator and perform any action just like a normal page.
iframe = page.frame_locator("#iframe-id")
iframe.locator("#button_inside_iframe").click()
iframe.fill("#username", "testuser")
iframe.click("#submit")
By using these methods, we can seamlessly interact with iframes in Playwright.
9. How do you handle file uploads in Playwright?
Answer: In Playwright, we can handle file uploads using the set_input_files() method. This allows us to programmatically attach files to file input elements.
We can upload a single file or multiple based on our requirements.
page.set_input_files("input[type='file']", "path/to/file.png")
page.set_input_files("input[type='file']", ["file1.png", "file2.jpg"])
If we need to reset the file input, we can pass an empty list [].
page.set_input_files("input[type='file']", [])
In some cases, we may need to wait for the upload process to finish before proceeding.
page.set_input_files("input[type='file']", "path-to-file-pdf")
# Wait for success message
page.wait_for_selector("#upload-success")
10. How do you handle file downloads in Playwright?
Answer: In Playwright, we can handle file downloads using the page.expect_download() method, which waits for a download event and allows us to save the file.
Downloading a file and saving it to a specific location:
with page.expect_download() as download_info:
# Click the download link/button
page.click("#download-button")
download = download_info.value
# Save to a custom location
download.save_as("path-to-file-pdf")
Getting the downloaded file path:
with page.expect_download() as download_info:
page.click("#download-link")
download = download_info.value
# Get the local path
print("File downloaded to:", download.path())
Waiting for download completion before proceeding:
with page.expect_download() as download_info:
page.click("#export-data")
download = download_info.value
# Ensures the file is fully downloaded
download.path()
By using expect_download(), Playwright allows us to efficiently automate file downloads while ensuring that the process completes successfully.
Performance & debugging interview questions
11. How do you perform API testing in Playwright?
Answer: In Playwright, we can perform API testing using the APIRequestContext class, which allows making HTTP requests directly from tests. This helps in validating API responses without interacting with the UI.
This is how we can send a GET request and validate the response.
response = page.request.get("<specify the base url>/data")
# Check HTTP status
assert response.status == 200
# Print response data
print(response.json())
Similarly, we can send a POST request with JSON data.
response = page.request.post(
"<specify the base url>/login",
data={"username": "testuser", "password": "securepass"},
)
assert response.status == 200
# Print response body
print(response.json())
Usually, we also have to send requests with headers and authentication.
response = page.request.get(
"<specify the base url>/protected",
headers={"Authorization": "Bearer my_token"}
)
assert response.status == 200
With Playwright’s built-in API testing feature, we can validate backend services, test API integrations, and mock responses without using tools like Postman.
12. How do you debug Playwright tests?
Answer: Playwright provides a built-in pause() method, which stops execution and opens the Playwright Inspector for manual interaction.
# Execution stops here, allowing manual inspection
page.pause()
👉 This lets us inspect elements, run commands, and interact with the page live.
By default, Playwright runs in headless mode. Running in headed mode allows us to visually observe test execution.
# Open browser with UI
browser = p.chromium.launch(headless=False)
We can enable tracing in Playwright to record test execution as a trace. It can be analysed later using the Playwright Trace Viewer.
context.tracing.start(screenshots=True, snapshots=True)
# Run test steps...
# Save trace for analysis
context.tracing.stop(path="trace.zip")
To open and inspect the trace:
npx playwright show-trace trace.zip
👉 The trace includes screenshots, network requests, console logs, and more.
13. How do you handle flaky tests in Playwright?
Answer: Flaky tests are a common challenge, and I tackle them with the following approach:
- Retries: I configure retries either in the Playwright config file or at the test level to automatically rerun failed tests.
- Waits: Instead of fixed timeouts, I use waitForSelector() and expect() assertions to handle dynamic elements.
- Tracing: When a test fails intermittently, I enable tracing (–trace=on-first-retry) to track issues.
- Backend Delays: If an API response is slow, I wait for specific requests using waitForResponse().
- Parallel Execution: When running tests in parallel, I ensure independent test data and avoid shared state issues.
Scenario-based Playwright interview questions
14. What challenges have you faced using Playwright, and how did you solve them?
Answer: Some common challenges I faced were:
- Handling dynamic elements: Solved by using the locator().wait_for() instead of explicit waits.
- Network request failures: I was able to manage by using mocked responses using route().
- Authentication issues: I backed up the session storage and reused it.
15. How would you automate a login test where the login button is disabled until valid inputs are entered?
Answer: In Playwright, we can automate this scenario by verifying the button’s enabled or disabled state before and after entering valid credentials. Here’s how:
from playwright.sync_api import sync_playwright, expect
def test_login_button_state():
with sync_playwright() as p:
browser = p.chromium.launch()
page = browser.new_page()
page.goto("<specify the base url>/login")
# Locate the username, password fields, and login button
username_input = page.locator("#username")
password_input = page.locator("#password")
login_button = page.locator("#login-button")
# Assert the login button is disabled initially
expect(login_button).to_be_disabled()
# Fill in valid username and password
username_input.fill("valid_username")
password_input.fill("valid_password")
# Assert the login button is enabled after entering valid inputs
expect(login_button).to_be_enabled()
# Optionally, click the login button to proceed
login_button.click()
16. How do you handle authentication pop-ups in Playwright?
Answer: We can use the browser.new_context() with credentials.
context = browser.new_context(http_credentials={"username": "user", "password": "pass"})
page = context.new_page()
page.goto("<specify the website url>")
17. How do you handle dynamic elements in Playwright?
Answer: Use locator.wait_for() to wait for an element before interacting.
page.locator("#dynamic-element").wait_for()
We can also use expect assertions:
from playwright.sync_api import expect
expect(page.locator("#dynamic-element")).to_be_visible()
18. How do you perform parallel testing in Playwright?
Answer: Use pytest with Playwright to run tests in parallel:
pytest --numprocesses=4
Or we can also use multiple browser contexts:
with sync_playwright() as p:
browser = p.chromium.launch()
context1 = browser.new_context()
context2 = browser.new_context()
page1 = context1.new_page()
page2 = context2.new_page()
19. How do you handle network interception and request modification in Playwright?
Answer: The simple way is to by calling the page.route() to mock responses:
def mock_response(route, request):
route.fulfill(status=200, body="{\"message\": \"Mocked response\"}")
page.route("**/api/data", mock_response)
20. How do you capture console logs in Playwright?
Answer: In Playwright, capturing console logs is essential for debugging. We can set up an event listener on the page object to listen for ‘console’ events. Here’s how to do it in Python:
from playwright.sync_api import sync_playwright
def handle_console_message(msg):
print(f"Console [{msg.type()}]: {msg.text()}")
with sync_playwright() as p:
browser = p.chromium.launch()
page = browser.new_page()
# Listen to console events
page.on("console", handle_console_message)
page.goto("<specify the website url>")
# Perform additional actions as needed
browser.close()
This tutorial covered essential Playwright interview questions with structured, practical answers. Let me know if you need more questions added. For this, don’t miss to subscribe our YouTube channel. 🚀