Problem – How to Zoom In/Out in Webdriver
In this tutorial, we’ll explore how to zoom in and out in Selenium Webdriver using Java. This functionality is crucial for responsive designs or capturing full-page screenshots. We’ll discuss two methods, compare their pros and cons, and guide you to choose the best approach for your automation needs.
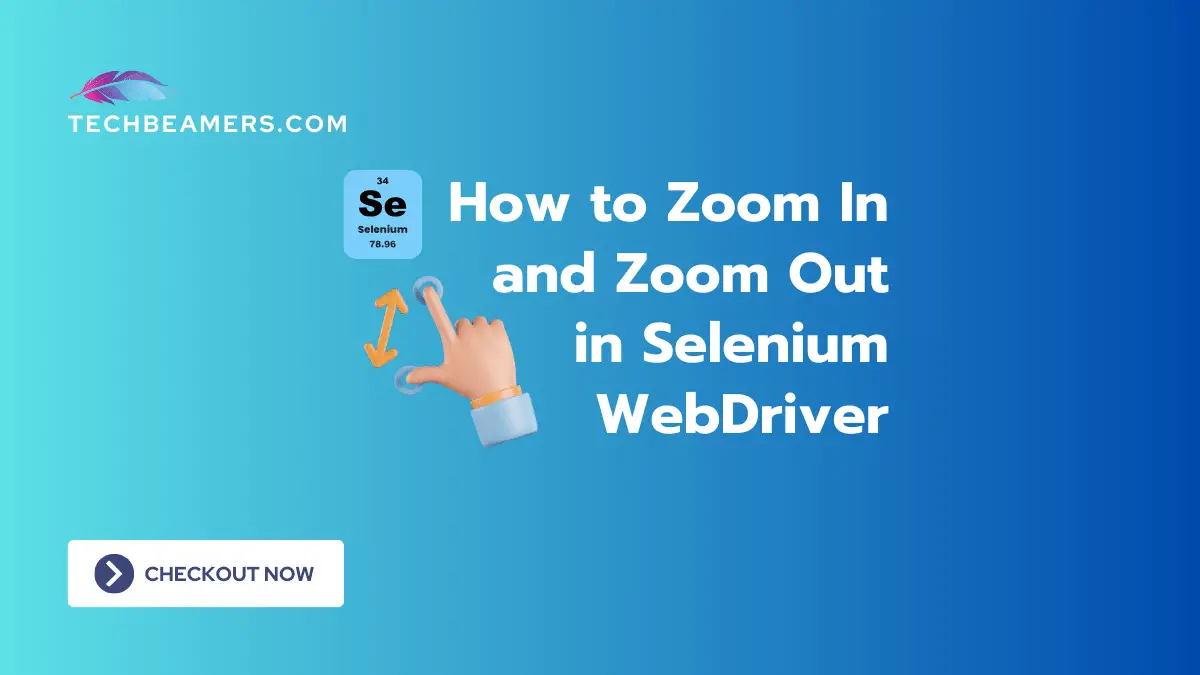
Prerequisites for Selenium Java
Before diving into the tutorial, ensure you have the following prerequisites:
Solutions for Zoom In / Zoom Out
Let’s start by setting up a basic Selenium WebDriver project. Create a new Java project in your favorite IDE (Eclipse, IntelliJ, etc.) and add the Selenium WebDriver library to your project’s build path.
Using JavaScript Executor
The first method involves using JavascriptExecutor to execute JavaScript code that adjusts the zoom level. Let’s create a Java class named ZoomInOutJS
to implement this method:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.JavascriptExecutor;
public class ZoomInOutJS {
public static void main(String[] args) {
// Set up the ChromeDriver
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
// Start the Chrome browser
WebDriver driver = new ChromeDriver();
// Open a website
driver.get("https://example.com");
// Zoom in the webpage
zoomIn(driver);
// Pause to see the zoom effect
pause(2000);
// Zoom out the webpage
zoomOut(driver);
// Close the browser
driver.quit();
}
// Function to zoom in
private static void zoomIn(WebDriver driver) {
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("document.body.style.zoom = '120%';");
}
// Function to zoom out
private static void zoomOut(WebDriver driver) {
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("document.body.style.zoom = '80%';");
}
// Function to pause the code
private static void pause(int ms) {
try {
Thread.sleep(ms);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
Pros:
- The code changes the zoom level directly using JavaScript, which can be precise.
- It allows for detailed control over how much to zoom in or out.
Cons:
- It might not work well with some page elements or layouts.
- Certain web pages may not display correctly when zoomed this way.
Using Browser Functionality
The second method involves using keyboard shortcuts to trigger the browser’s built-in functionality. Let’s create a Java class named ZoomInOutBrowser
to implement this method:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
import org.openqa.selenium.Keys;
public class ZoomInOutBrowser {
public static void main(String[] args) {
// Set up the ChromeDriver
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
// Start the Chrome browser
WebDriver driver = new ChromeDriver();
// Open a website
driver.get("https://example.com");
// Zooming in the browser window
zoomIn(driver);
// Pause to see the zoom effect
pause(2000);
// Zooming out the browser window
zoomOut(driver);
// Close the browser
driver.quit();
}
// Function to zoom in
private static void zoomIn(WebDriver driver) {
Actions act = new Actions(driver);
act.keyDown(Keys.CONTROL).sendKeys(Keys.ADD).keyUp(Keys.CONTROL).perform();
}
// Function to zoom out
private static void zoomOut(WebDriver driver) {
Actions act = new Actions(driver);
act.keyDown(Keys.CONTROL).sendKeys(Keys.SUBTRACT).keyUp(Keys.CONTROL).perform();
}
// Function to pause the code
private static void pause(int ms) {
try {
Thread.sleep(ms);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
Pros:
- This code uses the browser’s own zoom feature, which makes it easy to increase or reduce the view.
- It simulates how a real user would zoom, giving a realistic test experience.
Cons:
- The code might not work the same way in all browsers.
- It depends on keyboard shortcuts, which can be different in some browsers.
More Related Topics:
How to Generate Extent Report in Selenium with Python, Java, and C#
Cypress vs Selenium – Which One Should You be Using in 2024
How to Use Selenium IDE to Add Input-based Test Cases
When Selenium Click() not Working – What to do?
General Questions
Here are some of the common queries on this topic.
Q1: Why is zooming important in Selenium WebDriver?
A1: It is important to test how a website looks on different screen sizes. It helps ensure the site works well when the screen size is large or small.
Q2: Can I zoom to a specific percentage using these methods?
A2: Yes, you can set to any percentage you want by adjusting the values in the code.
Q3: Does browser zoom affect automated testing?
A3: Yes, it changes how elements appear on the page. Testing with different levels ensures the site works on all screen sizes.
Q4: Can I use these methods for other browsers?
A4: Yes, these methods can be used in any browser. Just make sure to set up the WebDriver for the browser you’re using.
Q5: How can I zoom in headless mode?
A5: These methods work in both regular and headless modes. Run your tests in headless mode, and zooming will still work.
Additional Resources:
Handle iFrame/iFrames in Selenium Webdriver
Use Selenium WebDriver Waits in Python
How to Locate Elements using Selenium Python
Before You Leave
You’ve explored two methods to zoom in/out on a webpage using Selenium WebDriver in Java. Each method has its pros and cons, and the choice depends on your specific testing requirements. Experiment with both approaches to determine which one aligns better with your automation needs.
TechBeamers will keep providing new tutorials in the near future. In the meantime, please keep reading, learning, and subscribe to our YouTube channel.
Happy testing!